Analyzing Veros#
Veros makes a very nice output that is relatively easy to analyze. However, it is relatively complex, organized data set, so this document has some pointers to help you get started making calculations using the data it provides.
See also: https://veros.readthedocs.io/en/latest/tutorial/analysis.html
NetCDF and xarray#
Veros outputs its files in Network Common Data Format NetCDF. This is a widely-used portable format that organizes data matrices by co-ordinates. If we do ncdump -h example.snapshot.nc
we get entries like:
dimensions:
xt = 200 ;
xu = 200 ;
yt = 4 ;
yu = 4 ;
zt = 90 ;
zw = 90 ;
tensor1 = 2 ;
tensor2 = 2 ;
Time = UNLIMITED ; // (30 currently)
variables:
...
double Time(Time) ;
string Time:long_name = "Time" ;
string Time:units = "days" ;
string Time:time_origin = "01-JAN-1900 00:00:00" ;
...
double u(Time, zt, yt, xu) ;
u:_FillValue = -1.e+18 ;
string u:long_name = "Zonal velocity" ;
string u:units = "m/s" ;
...
double temp(Time, zt, yt, xt) ;
temp:_FillValue = -1.e+18 ;
string temp:long_name = "Temperature" ;
string temp:units = "deg C" ;
...
So, there are x,y,z and Time dimensions, and each of the variables is assigned to one or more of those dimensions. For instance temp
is a 4-dimensional variable with the three spatial dimensions and the one time dimension, and represents the evolution of temperature in the model over time.
NetCDF files can be quite large and sometimes cannot be read into memory on one machine. Fortunately the libraries that are written around them are very clever, and allow only parts of the files to be read or written at a time. So in the above, it is entirely possible with the right software to just read into memory one time step of one of the variables, which in this case is 90x4x200 large. Xarray is a very powerful way to work with NetCDF-like data sets and allows very efficient operations on NetCDF files. It is heavily based on numpy, which many students have already used, and also borrows many concepts from pandas. To read a data set into python we can use xarray:
import xarray as xr
import matplotlib.pyplot as plt
import numpy as np
# %matplotlib widget
# This makes it so we don't automatically see data variables. However you can click on the
# icon next to the variable to see the values if desired. You don't need to do this; its just
# being done to make the notebook compact:
xr.set_options(display_expand_data=False, display_expand_coords=False, display_expand_attrs=False)
<xarray.core.options.set_options at 0x106c13370>
with xr.open_dataset('example.snapshot.nc') as ds:
ds = ds.isel(Time=1)
ds['vcenter'] = ds.v.rolling(yu=2).mean().swap_dims({'yu':'yt'})
ds['ucenter'] = ds.u.rolling(xu=2).mean().swap_dims({'xu':'xt'})
ds['abs_speed'] = (ds.ucenter**2 + ds.vcenter**2)**(0.5)
abs_speed = (ds.u.values**2 + ds.v.values**2)**(0.5)
ds['rough_speed'] = (('zt', 'yt', 'xt'), abs_speed)
ds = ds.isel(yt=1, yu=1)
fig, axs = plt.subplots(1, 3, sharex=True, sharey=True,
layout='constrained', subplot_kw={'facecolor': '0.7'},
figsize=(6, 4))
pc = axs[0].pcolormesh(ds.abs_speed, clim=[-1, 1], cmap='RdBu_r')
fig.colorbar(pc, extend='both', shrink=0.6, orientation='horizontal')
axs[0].set_title('speed: centered')
pc = axs[1].pcolormesh(ds.rough_speed, clim=[-1, 1], cmap='RdBu_r')
fig.colorbar(pc, extend='both', shrink=0.6, orientation='horizontal')
axs[1].set_title('speed: rough')
pc = axs[2].pcolormesh(ds.rough_speed - ds.abs_speed, clim=[-1/10, 1/10], cmap='RdBu_r')
fig.colorbar(pc, extend='both', shrink=0.6, orientation='horizontal')
axs[2].set_title('speed: difference')
axs[2].set_xlim([75, 125])
axs[2].set_ylim([20, 100])
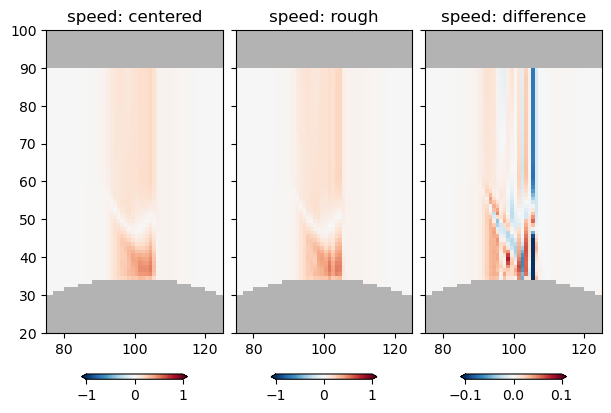
with xr.open_dataset('example.snapshot.nc') as ds:
display(ds)
<xarray.Dataset> Dimensions: (xt: 200, xu: 200, yt: 4, yu: 4, zt: 90, zw: 90, tensor1: 2, tensor2: 2, Time: 6) Coordinates: (9) Data variables: (12/33) dxt (xt) float64 ... dxu (xu) float64 ... dyt (yt) float64 ... dyu (yu) float64 ... dzt (zt) float64 ... dzw (zw) float64 ... ... ... kappaM (Time, zt, yt, xt) float64 ... kappaH (Time, zw, yt, xt) float64 ... surface_taux (Time, yt, xu) float64 ... surface_tauy (Time, yu, xt) float64 ... forc_rho_surface (Time, yt, xt) float64 ... psi (Time, yt, xt) float64 ... Attributes: (7)
- xt: 200
- xu: 200
- yt: 4
- yu: 4
- zt: 90
- zw: 90
- tensor1: 2
- tensor2: 2
- Time: 6
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- xu(xu)float64-149.6 -146.1 ... 149.6 153.1
- long_name :
- Zonal coordinate (U)
- units :
- km
array([-1.495829e+02, -1.461175e+02, -1.426563e+02, -1.391999e+02, -1.357491e+02, -1.323045e+02, -1.288671e+02, -1.254377e+02, -1.220174e+02, -1.186076e+02, -1.152094e+02, -1.118246e+02, -1.084547e+02, -1.051018e+02, -1.017680e+02, -9.845565e+01, -9.516740e+01, -9.190616e+01, -8.867512e+01, -8.547776e+01, -8.231785e+01, -7.919946e+01, -7.612691e+01, -7.310480e+01, -7.013796e+01, -6.723138e+01, -6.439016e+01, -6.161946e+01, -5.892438e+01, -5.630987e+01, -5.378062e+01, -5.134095e+01, -4.899467e+01, -4.674498e+01, -4.459441e+01, -4.254466e+01, -4.059661e+01, -3.875025e+01, -3.700472e+01, -3.535830e+01, -3.380848e+01, -3.235205e+01, -3.098520e+01, -2.970360e+01, -2.850258e+01, -2.737717e+01, -2.632228e+01, -2.533277e+01, -2.440350e+01, -2.352950e+01, -2.270595e+01, -2.192824e+01, -2.119205e+01, -2.049330e+01, -1.982824e+01, -1.919337e+01, -1.858552e+01, -1.800177e+01, -1.743949e+01, -1.689630e+01, -1.637003e+01, -1.585878e+01, -1.536080e+01, -1.487457e+01, -1.439871e+01, -1.393201e+01, -1.347337e+01, -1.302184e+01, -1.257657e+01, -1.213681e+01, -1.170189e+01, -1.127124e+01, -1.084434e+01, -1.042072e+01, -1.000000e+01, -9.600000e+00, -9.200000e+00, -8.800000e+00, -8.400000e+00, -8.000000e+00, -7.600000e+00, -7.200000e+00, -6.800000e+00, -6.400000e+00, -6.000000e+00, -5.600000e+00, -5.200000e+00, -4.800000e+00, -4.400000e+00, -4.000000e+00, -3.600000e+00, -3.200000e+00, -2.800000e+00, -2.400000e+00, -2.000000e+00, -1.600000e+00, -1.200000e+00, -8.000000e-01, -4.000000e-01, 2.910383e-14, 4.000000e-01, 8.000000e-01, 1.200000e+00, 1.600000e+00, 2.000000e+00, 2.400000e+00, 2.800000e+00, 3.200000e+00, 3.600000e+00, 4.000000e+00, 4.400000e+00, 4.800000e+00, 5.200000e+00, 5.600000e+00, 6.000000e+00, 6.400000e+00, 6.800000e+00, 7.200000e+00, 7.600000e+00, 8.000000e+00, 8.400000e+00, 8.800000e+00, 9.200000e+00, 9.600000e+00, 1.000000e+01, 1.042072e+01, 1.084434e+01, 1.127124e+01, 1.170189e+01, 1.213681e+01, 1.257657e+01, 1.302184e+01, 1.347337e+01, 1.393201e+01, 1.439871e+01, 1.487457e+01, 1.536080e+01, 1.585878e+01, 1.637003e+01, 1.689630e+01, 1.743949e+01, 1.800177e+01, 1.858552e+01, 1.919337e+01, 1.982824e+01, 2.049330e+01, 2.119205e+01, 2.192824e+01, 2.270595e+01, 2.352950e+01, 2.440350e+01, 2.533277e+01, 2.632228e+01, 2.737717e+01, 2.850258e+01, 2.970360e+01, 3.098520e+01, 3.235205e+01, 3.380848e+01, 3.535830e+01, 3.700472e+01, 3.875025e+01, 4.059661e+01, 4.254466e+01, 4.459441e+01, 4.674498e+01, 4.899467e+01, 5.134095e+01, 5.378062e+01, 5.630987e+01, 5.892438e+01, 6.161946e+01, 6.439016e+01, 6.723138e+01, 7.013796e+01, 7.310480e+01, 7.612691e+01, 7.919946e+01, 8.231785e+01, 8.547776e+01, 8.867512e+01, 9.190616e+01, 9.516740e+01, 9.845565e+01, 1.017680e+02, 1.051018e+02, 1.084547e+02, 1.118246e+02, 1.152094e+02, 1.186076e+02, 1.220174e+02, 1.254377e+02, 1.288671e+02, 1.323045e+02, 1.357491e+02, 1.391999e+02, 1.426563e+02, 1.461175e+02, 1.495829e+02, 1.530521e+02])
- yt(yt)float64-0.5 0.5 1.5 2.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array([-0.5, 0.5, 1.5, 2.5])
- yu(yu)float640.0 1.0 2.0 3.0
- long_name :
- Meridional coordinate (U)
- units :
- km
array([0., 1., 2., 3.])
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- zw(zw)float64-197.8 -195.6 -193.3 ... -2.222 0.0
- long_name :
- Vertical coordinate (W)
- units :
- m
- positive :
- up
array([-197.777778, -195.555556, -193.333333, -191.111111, -188.888889, -186.666667, -184.444444, -182.222222, -180. , -177.777778, -175.555556, -173.333333, -171.111111, -168.888889, -166.666667, -164.444444, -162.222222, -160. , -157.777778, -155.555556, -153.333333, -151.111111, -148.888889, -146.666667, -144.444444, -142.222222, -140. , -137.777778, -135.555556, -133.333333, -131.111111, -128.888889, -126.666667, -124.444444, -122.222222, -120. , -117.777778, -115.555556, -113.333333, -111.111111, -108.888889, -106.666667, -104.444444, -102.222222, -100. , -97.777778, -95.555556, -93.333333, -91.111111, -88.888889, -86.666667, -84.444444, -82.222222, -80. , -77.777778, -75.555556, -73.333333, -71.111111, -68.888889, -66.666667, -64.444444, -62.222222, -60. , -57.777778, -55.555556, -53.333333, -51.111111, -48.888889, -46.666667, -44.444444, -42.222222, -40. , -37.777778, -35.555556, -33.333333, -31.111111, -28.888889, -26.666667, -24.444444, -22.222222, -20. , -17.777778, -15.555556, -13.333333, -11.111111, -8.888889, -6.666667, -4.444444, -2.222222, 0. ])
- tensor1(tensor1)float640.0 1.0
- long_name :
- tensor1
- units :
array([0., 1.])
- tensor2(tensor2)float640.0 1.0
- long_name :
- tensor2
- units :
array([0., 1.])
- Time(Time)timedelta64[ns]01:00:00.000028800 ... 06:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array([ 3600000028800, 7199999971200, 10800000000000, 14400000028800, 17999999971200, 21600000000000], dtype='timedelta64[ns]')
- dxt(xt)float64...
- long_name :
- Zonal T-grid spacing
- units :
- m
[200 values with dtype=float64]
- dxu(xu)float64...
- long_name :
- Zonal U-grid spacing
- units :
- m
[200 values with dtype=float64]
- dyt(yt)float64...
- long_name :
- Meridional T-grid spacing
- units :
- m
[4 values with dtype=float64]
- dyu(yu)float64...
- long_name :
- Meridional U-grid spacing
- units :
- m
[4 values with dtype=float64]
- dzt(zt)float64...
- long_name :
- Vertical spacing (T)
- units :
- m
[90 values with dtype=float64]
- dzw(zw)float64...
- long_name :
- Vertical spacing (W)
- units :
- m
[90 values with dtype=float64]
- ht(yt, xt)float64...
- long_name :
- Total depth (T)
- units :
- m
[800 values with dtype=float64]
- hu(yt, xu)float64...
- long_name :
- Total depth (U)
- units :
- m
[800 values with dtype=float64]
- hv(yu, xt)float64...
- long_name :
- Total depth (V)
- units :
- m
[800 values with dtype=float64]
- beta(yt, xt)float64...
- long_name :
- Change of Coriolis freq.
- units :
- 1/(ms)
[800 values with dtype=float64]
- area_t(yt, xt)float64...
- long_name :
- Area of T-box
- units :
- m^2
[800 values with dtype=float64]
- area_u(yt, xu)float64...
- long_name :
- Area of U-box
- units :
- m^2
[800 values with dtype=float64]
- area_v(yu, xt)float64...
- long_name :
- Area of V-box
- units :
- m^2
[800 values with dtype=float64]
- rho(Time, zt, yt, xt)float64...
- long_name :
- Density
- units :
- kg/m^3
[432000 values with dtype=float64]
- prho(Time, zt, yt, xt)float64...
- long_name :
- Potential density
- units :
- kg/m^3
[432000 values with dtype=float64]
- int_drhodT(Time, zt, yt, xt)float64...
- long_name :
- Der. of dyn. enthalpy by temperature
- units :
- kg / (m^2 deg C)
[432000 values with dtype=float64]
- int_drhodS(Time, zt, yt, xt)float64...
- long_name :
- Der. of dyn. enthalpy by salinity
- units :
- kg / (m^2 g / kg)
[432000 values with dtype=float64]
- Nsqr(Time, zw, yt, xt)float64...
- long_name :
- Square of stability frequency
- units :
- 1/s^2
[432000 values with dtype=float64]
- Hd(Time, zt, yt, xt)float64...
- long_name :
- Dynamic enthalpy
- units :
- m^2/s^2
[432000 values with dtype=float64]
- temp(Time, zt, yt, xt)float64...
- long_name :
- Temperature
- units :
- deg C
[432000 values with dtype=float64]
- salt(Time, zt, yt, xt)float64...
- long_name :
- Salinity
- units :
- g/kg
[432000 values with dtype=float64]
- forc_temp_surface(Time, yt, xt)float64...
- long_name :
- Surface temperature flux
- units :
- m deg C/s
[4800 values with dtype=float64]
- forc_salt_surface(Time, yt, xt)float64...
- long_name :
- Surface salinity flux
- units :
- m g/s kg
[4800 values with dtype=float64]
- u(Time, zt, yt, xu)float64...
- long_name :
- Zonal velocity
- units :
- m/s
[432000 values with dtype=float64]
- v(Time, zt, yu, xt)float64...
- long_name :
- Meridional velocity
- units :
- m/s
[432000 values with dtype=float64]
- w(Time, zw, yt, xt)float64...
- long_name :
- Vertical velocity
- units :
- m/s
[432000 values with dtype=float64]
- p_hydro(Time, zt, yt, xt)float64...
- long_name :
- Hydrostatic pressure
- units :
- m^2/s^2
[432000 values with dtype=float64]
- kappaM(Time, zt, yt, xt)float64...
- long_name :
- Vertical viscosity
- units :
- m^2/s
[432000 values with dtype=float64]
- kappaH(Time, zw, yt, xt)float64...
- long_name :
- Vertical diffusivity
- units :
- m^2/s
[432000 values with dtype=float64]
- surface_taux(Time, yt, xu)float64...
- long_name :
- Surface wind stress
- units :
- N/m^2
[4800 values with dtype=float64]
- surface_tauy(Time, yu, xt)float64...
- long_name :
- Surface wind stress
- units :
- N/m^2
[4800 values with dtype=float64]
- forc_rho_surface(Time, yt, xt)float64...
- long_name :
- Surface density flux
- units :
- kg / (m^2 s)
[4800 values with dtype=float64]
- psi(Time, yt, xt)float64...
- long_name :
- Surface pressure
- units :
- m^2/s^2
[4800 values with dtype=float64]
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- xuPandasIndex
PandasIndex(Float64Index([ -149.5829291388837, -146.11747691514034, -142.656286565749, -139.19994298483698, -135.74910906034242, -132.3045354026015, -128.86707108785163, -125.43767546229275, -122.0174310337388, -118.60755745098363, ... 122.01743103373877, 125.43767546229269, 128.86707108785154, 132.3045354026014, 135.74910906034236, 139.19994298483692, 142.65628656574899, 146.11747691514034, 149.5829291388837, 153.05212756523898], dtype='float64', name='xu', length=200))
- ytPandasIndex
PandasIndex(Float64Index([-0.5, 0.5, 1.5, 2.5], dtype='float64', name='yt'))
- yuPandasIndex
PandasIndex(Float64Index([0.0, 1.0, 2.0, 3.0], dtype='float64', name='yu'))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
- zwPandasIndex
PandasIndex(Float64Index([-197.77777777777814, -195.5555555555559, -193.33333333333368, -191.11111111111148, -188.88888888888926, -186.66666666666703, -184.4444444444448, -182.2222222222226, -180.00000000000037, -177.77777777777814, -175.5555555555559, -173.3333333333337, -171.11111111111148, -168.88888888888926, -166.66666666666703, -164.4444444444448, -162.2222222222226, -160.00000000000037, -157.77777777777814, -155.55555555555594, -153.3333333333337, -151.11111111111148, -148.88888888888926, -146.66666666666703, -144.44444444444483, -142.2222222222226, -140.00000000000037, -137.77777777777817, -135.55555555555594, -133.3333333333337, -131.11111111111148, -128.88888888888926, -126.66666666666703, -124.4444444444448, -122.22222222222257, -120.00000000000034, -117.77777777777811, -115.55555555555588, -113.33333333333366, -111.11111111111143, -108.8888888888892, -106.66666666666697, -104.44444444444474, -102.22222222222251, -100.00000000000028, -97.77777777777806, -95.55555555555583, -93.3333333333336, -91.11111111111137, -88.88888888888914, -86.66666666666691, -84.44444444444468, -82.22222222222246, -80.00000000000023, -77.777777777778, -75.55555555555577, -73.33333333333354, -71.11111111111131, -68.88888888888908, -66.66666666666686, -64.44444444444463, -62.2222222222224, -60.00000000000017, -57.77777777777794, -55.55555555555571, -53.333333333333485, -51.111111111111256, -48.88888888888903, -46.6666666666668, -44.44444444444457, -42.22222222222234, -40.000000000000114, -37.777777777777885, -35.55555555555566, -33.33333333333343, -31.1111111111112, -28.88888888888897, -26.666666666666742, -24.444444444444514, -22.222222222222285, -20.000000000000057, -17.77777777777783, -15.5555555555556, -13.333333333333371, -11.111111111111143, -8.888888888888914, -6.666666666666686, -4.444444444444457, -2.2222222222222285, 0.0], dtype='float64', name='zw'))
- tensor1PandasIndex
PandasIndex(Float64Index([0.0, 1.0], dtype='float64', name='tensor1'))
- tensor2PandasIndex
PandasIndex(Float64Index([0.0, 1.0], dtype='float64', name='tensor2'))
- TimePandasIndex
PandasIndex(TimedeltaIndex(['0 days 01:00:00.000028800', '0 days 01:59:59.999971200', '0 days 03:00:00', '0 days 04:00:00.000028800', '0 days 04:59:59.999971200', '0 days 06:00:00'], dtype='timedelta64[ns]', name='Time', freq=None))
- date_created :
- 2023-09-07T15:00:26.245784
- veros_version :
- 1.5.0
- setup_identifier :
- example
- setup_description :
- setup_settings :
- {"identifier": "example", "description": "", "nx": 200, "ny": 4, "nz": 90, "dt_mom": 20.0, "dt_tracer": 20.0, "runlen": 22320.0, "AB_eps": 0.1, "x_origin": -149582.92913888372, "y_origin": 0.0, "pi": 3.141592653589793, "radius": 6370000.0, "degtom": 111177.4733520388, "omega": 7.292123516990375e-05, "rho_0": 1024.0, "grav": 9.81, "coord_degree": false, "enable_cyclic_x": false, "eq_of_state_type": 1, "enable_implicit_vert_friction": true, "enable_explicit_vert_friction": false, "enable_hor_friction": true, "enable_hor_diffusion": true, "enable_biharmonic_friction": false, "enable_biharmonic_mixing": false, "enable_hor_friction_cos_scaling": false, "enable_ray_friction": false, "enable_bottom_friction": false, "enable_bottom_friction_var": false, "enable_quadratic_bottom_friction": true, "enable_tempsalt_sources": false, "enable_momentum_sources": false, "enable_superbee_advection": false, "enable_conserve_energy": true, "enable_store_bottom_friction_tke": false, "enable_store_cabbeling_heat": false, "enable_noslip_lateral": false, "enable_streamfunction": false, "A_h": 10.0, "K_h": 10.0, "r_ray": 0.0, "r_bot": 0.0, "r_quad_bot": 0.001, "hor_friction_cosPower": 1.0, "A_hbi": 0.0, "K_hbi": 0.0, "biharmonic_friction_cosPower": 0.0, "kappaH_0": 0.0004, "kappaM_0": 0.0004, "enable_neutral_diffusion": false, "enable_skew_diffusion": false, "enable_TEM_friction": false, "K_iso_0": 0.0, "K_iso_steep": 0.0, "K_gm_0": 0.0, "iso_dslope": 0.0008, "iso_slopec": 0.001, "enable_idemix": false, "tau_v": 172800.0, "tau_h": 1296000.0, "gamma": 1.57, "jstar": 5.0, "mu0": 0.3333333333333333, "enable_idemix_hor_diffusion": false, "enable_eke_diss_bottom": false, "enable_eke_diss_surfbot": false, "eke_diss_surfbot_frac": 1.0, "enable_idemix_superbee_advection": false, "enable_idemix_upwind_advection": false, "enable_tke": false, "c_k": 0.1, "c_eps": 0.7, "alpha_tke": 1.0, "mxl_min": 1e-12, "kappaM_min": 0.0, "kappaM_max": 100.0, "tke_mxl_choice": 1, "enable_tke_superbee_advection": false, "enable_tke_upwind_advection": false, "enable_tke_hor_diffusion": false, "K_h_tke": 2000.0, "enable_eke": false, "eke_lmin": 100.0, "eke_c_k": 1.0, "eke_cross": 1.0, "eke_crhin": 1.0, "eke_c_eps": 1.0, "eke_k_max": 10000.0, "alpha_eke": 1.0, "enable_eke_superbee_advection": false, "enable_eke_upwind_advection": false, "enable_eke_isopycnal_diffusion": false, "restart_input_filename": null, "restart_output_filename": "{identifier}_{itt:0>4d}.restart.h5", "restart_frequency": 0.0, "kappaH_min": 0.0, "enable_kappaH_profile": false, "enable_Prandtl_tke": true, "Prandtl_tke0": 10.0}
- setup_file :
- /Users/jklymak/Dropbox/Teaching/Eos431Phy441/site/computing/example.py
- setup_code :
- #!/usr/bin/env python """ """ __VEROS_VERSION__ = '1.5.0' if __name__ == "__main__": raise RuntimeError( "Veros setups cannot be executed directly. " f"Try `veros run {__file__}` instead." ) # -- end of auto-generated header, original file below -- from veros import VerosSetup, veros_routine from veros.variables import allocate, Variable from veros.distributed import global_min, global_max, proc_index_to_rank, proc_rank_to_index from veros.core.operators import numpy as npx, update, at from veros.tools.setup import get_stretched_grid_steps from veros import runtime_settings as rs, runtime_state as rst class ChannelSetup(VerosSetup): """A model using cartesian coordinates in cyclic channel Wind forcing over a channel; no buoyancy """ @veros_routine def set_parameter(self, state): settings = state.settings settings.identifier = "example" settings.nx, settings.ny, settings.nz = 200, 4, 90 settings.dt_mom = 20 settings.dt_tracer = settings.dt_mom settings.runlen = 3600 * 6.2 settings.x_origin = 0.0 settings.y_origin = 0.0 settings.coord_degree = False settings.enable_cyclic_x = False settings.enable_neutral_diffusion = False settings.enable_hor_friction = True settings.enable_hor_diffusion = True settings.A_h = 10 settings.K_h = settings.A_h settings.enable_hor_friction_cos_scaling = False settings.hor_friction_cosPower = 1 settings.enable_quadratic_bottom_friction = True settings.r_quad_bot = 1e-3 settings.enable_implicit_vert_friction = True settings.enable_explicit_vert_friction = False settings.kappaM_0 = 4e-4 settings.kappaH_0 = 4e-4 settings.enable_streamfunction = False settings.enable_idemix = False settings.eq_of_state_type = 1 @veros_routine(dist_safe=False, local_variables=['dxt', 'dyt', 'dzt']) def set_grid(self, state): vs = state.variables settings = state.settings proc_idx = proc_rank_to_index(rst.proc_rank) print(proc_idx) ddz = 200 / settings.nz print(npx.shape(vs.dxt)) if False: vs.dxt = update(vs.dxt, at[...], 400) if True: for i in range(int(settings.nx / 3), -1, -1): vs.dxt = update(vs.dxt, at[i], vs.dxt[i+1] * 1.05) for i in range(int(2*settings.nx / 3)-1, settings.nx+4): vs.dxt = update(vs.dxt, at[i], vs.dxt[i-1] * 1.05) dx = get_stretched_grid_steps(102-25, 150e3, 400) vs.dxt = update(vs.dxt, at[...], npx.hstack((dx[::-1], npx.ones(50)*400, dx))) vs.dyt = update(vs.dyt, at[...], 1000) vs.dzt = update(vs.dzt, at[...], ddz) @veros_routine def set_coriolis(self, state): vs = state.variables # settings = state.settings vs.coriolis_t = update( vs.coriolis_t, at[...], 0e-4 ) @veros_routine(dist_safe=False, local_variables=['kbot', 'xt']) def set_topography(self, state): vs = state.variables # depth of deepest cell. 0 is land, 1 is deepest # nz is shallowest.. vs.kbot = update(vs.kbot, at[...], 1) kbot = npx.ones_like(vs.kbot[:, 0]) x = vs.xt x = x - npx.mean(x) kbot += npx.floor(35*npx.exp(-(x/25000)**2)).astype("int") for j in range(state.settings.ny+1): vs.kbot = update(vs.kbot, at[:, j], kbot) vs.kbot = update(vs.kbot, at[:, 0], 0) vs.kbot = update(vs.kbot, at[0, :], 0) @veros_routine(dist_safe=False, local_variables=['xu', 'xt', 'temp']) def set_initial_conditions(self, state): vs = state.variables settings = state.settings nx = settings.nx tbot = 10 ttop = 14 inx = npx.nonzero(vs.xt > npx.mean(vs.xt))[0] with settings.unlock(): settings.x_origin = -npx.mean(vs.xt) vs.xt = update(vs.xt, at[...], vs.xt+settings.x_origin) vs.xu = update(vs.xu, at[...], vs.xu+settings.x_origin) vs.temp = update(vs.temp, at[:inx[0], :, :, :], tbot) vs.temp = update(vs.temp, at[inx[0]:, :, :, :], ttop) vs.temp = update(vs.temp, at[:, :, :30, :], tbot) vs.temp = update(vs.temp, at[:, :, settings.nz-30:, :], ttop) @veros_routine def set_forcing(self, state): pass # vs = state.variables # vs.forc_temp_surface = vs.t_rest * (vs.t_star - vs.temp[:, :, -1, vs.tau]) @veros_routine def set_diagnostics(self, state): settings = state.settings diagnostics = state.diagnostics diagnostics["snapshot"].output_frequency = 7200 / 2 @veros_routine def after_timestep(self, state): pass
Note that in the above, you can click on the triangles to expand or collapse the Data variables
or the Coordinates
.
As you can see the xarray data set has the same structure as the NetCDF file. In addition it has the concept of Coordinates
, which are special variables that correspond to the dimensions.
Each Dataset
is composed of one or more DataArrays
which often share coordinates and dimensions with other DataArrays
in the Dataset
.
So in the above temp
and salt
are DataArray
s representing temperature and salinity in the model, and they share the dimensions Time, zt, xt
.
display(ds.temp)
display(ds.salt)
<xarray.DataArray 'temp' (Time: 6, zt: 90, yt: 4, xt: 200)> [432000 values with dtype=float64] Coordinates: (4) Attributes: (2)
- Time: 6
- zt: 90
- yt: 4
- xt: 200
- ...
[432000 values with dtype=float64]
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- yt(yt)float64-0.5 0.5 1.5 2.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array([-0.5, 0.5, 1.5, 2.5])
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- Time(Time)timedelta64[ns]01:00:00.000028800 ... 06:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array([ 3600000028800, 7199999971200, 10800000000000, 14400000028800, 17999999971200, 21600000000000], dtype='timedelta64[ns]')
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- ytPandasIndex
PandasIndex(Float64Index([-0.5, 0.5, 1.5, 2.5], dtype='float64', name='yt'))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
- TimePandasIndex
PandasIndex(TimedeltaIndex(['0 days 01:00:00.000028800', '0 days 01:59:59.999971200', '0 days 03:00:00', '0 days 04:00:00.000028800', '0 days 04:59:59.999971200', '0 days 06:00:00'], dtype='timedelta64[ns]', name='Time', freq=None))
- long_name :
- Temperature
- units :
- deg C
<xarray.DataArray 'salt' (Time: 6, zt: 90, yt: 4, xt: 200)> [432000 values with dtype=float64] Coordinates: (4) Attributes: (2)
- Time: 6
- zt: 90
- yt: 4
- xt: 200
- ...
[432000 values with dtype=float64]
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- yt(yt)float64-0.5 0.5 1.5 2.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array([-0.5, 0.5, 1.5, 2.5])
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- Time(Time)timedelta64[ns]01:00:00.000028800 ... 06:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array([ 3600000028800, 7199999971200, 10800000000000, 14400000028800, 17999999971200, 21600000000000], dtype='timedelta64[ns]')
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- ytPandasIndex
PandasIndex(Float64Index([-0.5, 0.5, 1.5, 2.5], dtype='float64', name='yt'))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
- TimePandasIndex
PandasIndex(TimedeltaIndex(['0 days 01:00:00.000028800', '0 days 01:59:59.999971200', '0 days 03:00:00', '0 days 04:00:00.000028800', '0 days 04:59:59.999971200', '0 days 06:00:00'], dtype='timedelta64[ns]', name='Time', freq=None))
- long_name :
- Salinity
- units :
- g/kg
Veros coordinates#
The coordinates above are all doubled except for Time
, e.g. there is a pair xt
and xu
. This is because Veros is solved on what is called an Arakawa C-Grid, with the velocities specified on the edges of grid cells that have temperature, salinity, pressure, and other tracers at their centre. See Veros Model Domain for more details, but the diagram looks like:
So, in the above, the variable u
has dimensions (Time, zt, yt, xu)
, v
has dimensions (time, zt, yu, xt)
and temp
has dimensions (Time, zt, yt, xt)
. The vertical velocity w
has dimensions (Time, zw, yt, xt)
.
Selecting data#
The data set above is probably not too big to fit in memory, but it still may be preferable to select subsets of the data. Xarray makes this realtively straight forward.
Selecting data by dimension index: isel
#
The method isel
means “index selection”. Suppose I want the 3rd timestep, and I only want a slice of data at the first value of y, then I can use isel
and reduce all the variables at the same time:
with xr.open_dataset('example.snapshot.nc') as ds:
ds = ds.isel(yt=0, yu=0, Time=2)
display(ds)
<xarray.Dataset> Dimensions: (xt: 200, xu: 200, zt: 90, zw: 90, tensor1: 2, tensor2: 2) Coordinates: (9) Data variables: (12/33) dxt (xt) float64 ... dxu (xu) float64 ... dyt float64 ... dyu float64 ... dzt (zt) float64 ... dzw (zw) float64 ... ... ... kappaM (zt, xt) float64 ... kappaH (zw, xt) float64 ... surface_taux (xu) float64 ... surface_tauy (xt) float64 ... forc_rho_surface (xt) float64 ... psi (xt) float64 ... Attributes: (7)
- xt: 200
- xu: 200
- zt: 90
- zw: 90
- tensor1: 2
- tensor2: 2
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- xu(xu)float64-149.6 -146.1 ... 149.6 153.1
- long_name :
- Zonal coordinate (U)
- units :
- km
array([-1.495829e+02, -1.461175e+02, -1.426563e+02, -1.391999e+02, -1.357491e+02, -1.323045e+02, -1.288671e+02, -1.254377e+02, -1.220174e+02, -1.186076e+02, -1.152094e+02, -1.118246e+02, -1.084547e+02, -1.051018e+02, -1.017680e+02, -9.845565e+01, -9.516740e+01, -9.190616e+01, -8.867512e+01, -8.547776e+01, -8.231785e+01, -7.919946e+01, -7.612691e+01, -7.310480e+01, -7.013796e+01, -6.723138e+01, -6.439016e+01, -6.161946e+01, -5.892438e+01, -5.630987e+01, -5.378062e+01, -5.134095e+01, -4.899467e+01, -4.674498e+01, -4.459441e+01, -4.254466e+01, -4.059661e+01, -3.875025e+01, -3.700472e+01, -3.535830e+01, -3.380848e+01, -3.235205e+01, -3.098520e+01, -2.970360e+01, -2.850258e+01, -2.737717e+01, -2.632228e+01, -2.533277e+01, -2.440350e+01, -2.352950e+01, -2.270595e+01, -2.192824e+01, -2.119205e+01, -2.049330e+01, -1.982824e+01, -1.919337e+01, -1.858552e+01, -1.800177e+01, -1.743949e+01, -1.689630e+01, -1.637003e+01, -1.585878e+01, -1.536080e+01, -1.487457e+01, -1.439871e+01, -1.393201e+01, -1.347337e+01, -1.302184e+01, -1.257657e+01, -1.213681e+01, -1.170189e+01, -1.127124e+01, -1.084434e+01, -1.042072e+01, -1.000000e+01, -9.600000e+00, -9.200000e+00, -8.800000e+00, -8.400000e+00, -8.000000e+00, -7.600000e+00, -7.200000e+00, -6.800000e+00, -6.400000e+00, -6.000000e+00, -5.600000e+00, -5.200000e+00, -4.800000e+00, -4.400000e+00, -4.000000e+00, -3.600000e+00, -3.200000e+00, -2.800000e+00, -2.400000e+00, -2.000000e+00, -1.600000e+00, -1.200000e+00, -8.000000e-01, -4.000000e-01, 2.910383e-14, 4.000000e-01, 8.000000e-01, 1.200000e+00, 1.600000e+00, 2.000000e+00, 2.400000e+00, 2.800000e+00, 3.200000e+00, 3.600000e+00, 4.000000e+00, 4.400000e+00, 4.800000e+00, 5.200000e+00, 5.600000e+00, 6.000000e+00, 6.400000e+00, 6.800000e+00, 7.200000e+00, 7.600000e+00, 8.000000e+00, 8.400000e+00, 8.800000e+00, 9.200000e+00, 9.600000e+00, 1.000000e+01, 1.042072e+01, 1.084434e+01, 1.127124e+01, 1.170189e+01, 1.213681e+01, 1.257657e+01, 1.302184e+01, 1.347337e+01, 1.393201e+01, 1.439871e+01, 1.487457e+01, 1.536080e+01, 1.585878e+01, 1.637003e+01, 1.689630e+01, 1.743949e+01, 1.800177e+01, 1.858552e+01, 1.919337e+01, 1.982824e+01, 2.049330e+01, 2.119205e+01, 2.192824e+01, 2.270595e+01, 2.352950e+01, 2.440350e+01, 2.533277e+01, 2.632228e+01, 2.737717e+01, 2.850258e+01, 2.970360e+01, 3.098520e+01, 3.235205e+01, 3.380848e+01, 3.535830e+01, 3.700472e+01, 3.875025e+01, 4.059661e+01, 4.254466e+01, 4.459441e+01, 4.674498e+01, 4.899467e+01, 5.134095e+01, 5.378062e+01, 5.630987e+01, 5.892438e+01, 6.161946e+01, 6.439016e+01, 6.723138e+01, 7.013796e+01, 7.310480e+01, 7.612691e+01, 7.919946e+01, 8.231785e+01, 8.547776e+01, 8.867512e+01, 9.190616e+01, 9.516740e+01, 9.845565e+01, 1.017680e+02, 1.051018e+02, 1.084547e+02, 1.118246e+02, 1.152094e+02, 1.186076e+02, 1.220174e+02, 1.254377e+02, 1.288671e+02, 1.323045e+02, 1.357491e+02, 1.391999e+02, 1.426563e+02, 1.461175e+02, 1.495829e+02, 1.530521e+02])
- yt()float64-0.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array(-0.5)
- yu()float640.0
- long_name :
- Meridional coordinate (U)
- units :
- km
array(0.)
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- zw(zw)float64-197.8 -195.6 -193.3 ... -2.222 0.0
- long_name :
- Vertical coordinate (W)
- units :
- m
- positive :
- up
array([-197.777778, -195.555556, -193.333333, -191.111111, -188.888889, -186.666667, -184.444444, -182.222222, -180. , -177.777778, -175.555556, -173.333333, -171.111111, -168.888889, -166.666667, -164.444444, -162.222222, -160. , -157.777778, -155.555556, -153.333333, -151.111111, -148.888889, -146.666667, -144.444444, -142.222222, -140. , -137.777778, -135.555556, -133.333333, -131.111111, -128.888889, -126.666667, -124.444444, -122.222222, -120. , -117.777778, -115.555556, -113.333333, -111.111111, -108.888889, -106.666667, -104.444444, -102.222222, -100. , -97.777778, -95.555556, -93.333333, -91.111111, -88.888889, -86.666667, -84.444444, -82.222222, -80. , -77.777778, -75.555556, -73.333333, -71.111111, -68.888889, -66.666667, -64.444444, -62.222222, -60. , -57.777778, -55.555556, -53.333333, -51.111111, -48.888889, -46.666667, -44.444444, -42.222222, -40. , -37.777778, -35.555556, -33.333333, -31.111111, -28.888889, -26.666667, -24.444444, -22.222222, -20. , -17.777778, -15.555556, -13.333333, -11.111111, -8.888889, -6.666667, -4.444444, -2.222222, 0. ])
- tensor1(tensor1)float640.0 1.0
- long_name :
- tensor1
- units :
array([0., 1.])
- tensor2(tensor2)float640.0 1.0
- long_name :
- tensor2
- units :
array([0., 1.])
- Time()timedelta64[ns]03:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array(10800000000000, dtype='timedelta64[ns]')
- dxt(xt)float64...
- long_name :
- Zonal T-grid spacing
- units :
- m
[200 values with dtype=float64]
- dxu(xu)float64...
- long_name :
- Zonal U-grid spacing
- units :
- m
[200 values with dtype=float64]
- dyt()float64...
- long_name :
- Meridional T-grid spacing
- units :
- m
[1 values with dtype=float64]
- dyu()float64...
- long_name :
- Meridional U-grid spacing
- units :
- m
[1 values with dtype=float64]
- dzt(zt)float64...
- long_name :
- Vertical spacing (T)
- units :
- m
[90 values with dtype=float64]
- dzw(zw)float64...
- long_name :
- Vertical spacing (W)
- units :
- m
[90 values with dtype=float64]
- ht(xt)float64...
- long_name :
- Total depth (T)
- units :
- m
[200 values with dtype=float64]
- hu(xu)float64...
- long_name :
- Total depth (U)
- units :
- m
[200 values with dtype=float64]
- hv(xt)float64...
- long_name :
- Total depth (V)
- units :
- m
[200 values with dtype=float64]
- beta(xt)float64...
- long_name :
- Change of Coriolis freq.
- units :
- 1/(ms)
[200 values with dtype=float64]
- area_t(xt)float64...
- long_name :
- Area of T-box
- units :
- m^2
[200 values with dtype=float64]
- area_u(xu)float64...
- long_name :
- Area of U-box
- units :
- m^2
[200 values with dtype=float64]
- area_v(xt)float64...
- long_name :
- Area of V-box
- units :
- m^2
[200 values with dtype=float64]
- rho(zt, xt)float64...
- long_name :
- Density
- units :
- kg/m^3
[18000 values with dtype=float64]
- prho(zt, xt)float64...
- long_name :
- Potential density
- units :
- kg/m^3
[18000 values with dtype=float64]
- int_drhodT(zt, xt)float64...
- long_name :
- Der. of dyn. enthalpy by temperature
- units :
- kg / (m^2 deg C)
[18000 values with dtype=float64]
- int_drhodS(zt, xt)float64...
- long_name :
- Der. of dyn. enthalpy by salinity
- units :
- kg / (m^2 g / kg)
[18000 values with dtype=float64]
- Nsqr(zw, xt)float64...
- long_name :
- Square of stability frequency
- units :
- 1/s^2
[18000 values with dtype=float64]
- Hd(zt, xt)float64...
- long_name :
- Dynamic enthalpy
- units :
- m^2/s^2
[18000 values with dtype=float64]
- temp(zt, xt)float64...
- long_name :
- Temperature
- units :
- deg C
[18000 values with dtype=float64]
- salt(zt, xt)float64...
- long_name :
- Salinity
- units :
- g/kg
[18000 values with dtype=float64]
- forc_temp_surface(xt)float64...
- long_name :
- Surface temperature flux
- units :
- m deg C/s
[200 values with dtype=float64]
- forc_salt_surface(xt)float64...
- long_name :
- Surface salinity flux
- units :
- m g/s kg
[200 values with dtype=float64]
- u(zt, xu)float64...
- long_name :
- Zonal velocity
- units :
- m/s
[18000 values with dtype=float64]
- v(zt, xt)float64...
- long_name :
- Meridional velocity
- units :
- m/s
[18000 values with dtype=float64]
- w(zw, xt)float64...
- long_name :
- Vertical velocity
- units :
- m/s
[18000 values with dtype=float64]
- p_hydro(zt, xt)float64...
- long_name :
- Hydrostatic pressure
- units :
- m^2/s^2
[18000 values with dtype=float64]
- kappaM(zt, xt)float64...
- long_name :
- Vertical viscosity
- units :
- m^2/s
[18000 values with dtype=float64]
- kappaH(zw, xt)float64...
- long_name :
- Vertical diffusivity
- units :
- m^2/s
[18000 values with dtype=float64]
- surface_taux(xu)float64...
- long_name :
- Surface wind stress
- units :
- N/m^2
[200 values with dtype=float64]
- surface_tauy(xt)float64...
- long_name :
- Surface wind stress
- units :
- N/m^2
[200 values with dtype=float64]
- forc_rho_surface(xt)float64...
- long_name :
- Surface density flux
- units :
- kg / (m^2 s)
[200 values with dtype=float64]
- psi(xt)float64...
- long_name :
- Surface pressure
- units :
- m^2/s^2
[200 values with dtype=float64]
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- xuPandasIndex
PandasIndex(Float64Index([ -149.5829291388837, -146.11747691514034, -142.656286565749, -139.19994298483698, -135.74910906034242, -132.3045354026015, -128.86707108785163, -125.43767546229275, -122.0174310337388, -118.60755745098363, ... 122.01743103373877, 125.43767546229269, 128.86707108785154, 132.3045354026014, 135.74910906034236, 139.19994298483692, 142.65628656574899, 146.11747691514034, 149.5829291388837, 153.05212756523898], dtype='float64', name='xu', length=200))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
- zwPandasIndex
PandasIndex(Float64Index([-197.77777777777814, -195.5555555555559, -193.33333333333368, -191.11111111111148, -188.88888888888926, -186.66666666666703, -184.4444444444448, -182.2222222222226, -180.00000000000037, -177.77777777777814, -175.5555555555559, -173.3333333333337, -171.11111111111148, -168.88888888888926, -166.66666666666703, -164.4444444444448, -162.2222222222226, -160.00000000000037, -157.77777777777814, -155.55555555555594, -153.3333333333337, -151.11111111111148, -148.88888888888926, -146.66666666666703, -144.44444444444483, -142.2222222222226, -140.00000000000037, -137.77777777777817, -135.55555555555594, -133.3333333333337, -131.11111111111148, -128.88888888888926, -126.66666666666703, -124.4444444444448, -122.22222222222257, -120.00000000000034, -117.77777777777811, -115.55555555555588, -113.33333333333366, -111.11111111111143, -108.8888888888892, -106.66666666666697, -104.44444444444474, -102.22222222222251, -100.00000000000028, -97.77777777777806, -95.55555555555583, -93.3333333333336, -91.11111111111137, -88.88888888888914, -86.66666666666691, -84.44444444444468, -82.22222222222246, -80.00000000000023, -77.777777777778, -75.55555555555577, -73.33333333333354, -71.11111111111131, -68.88888888888908, -66.66666666666686, -64.44444444444463, -62.2222222222224, -60.00000000000017, -57.77777777777794, -55.55555555555571, -53.333333333333485, -51.111111111111256, -48.88888888888903, -46.6666666666668, -44.44444444444457, -42.22222222222234, -40.000000000000114, -37.777777777777885, -35.55555555555566, -33.33333333333343, -31.1111111111112, -28.88888888888897, -26.666666666666742, -24.444444444444514, -22.222222222222285, -20.000000000000057, -17.77777777777783, -15.5555555555556, -13.333333333333371, -11.111111111111143, -8.888888888888914, -6.666666666666686, -4.444444444444457, -2.2222222222222285, 0.0], dtype='float64', name='zw'))
- tensor1PandasIndex
PandasIndex(Float64Index([0.0, 1.0], dtype='float64', name='tensor1'))
- tensor2PandasIndex
PandasIndex(Float64Index([0.0, 1.0], dtype='float64', name='tensor2'))
- date_created :
- 2023-09-07T15:00:26.245784
- veros_version :
- 1.5.0
- setup_identifier :
- example
- setup_description :
- setup_settings :
- {"identifier": "example", "description": "", "nx": 200, "ny": 4, "nz": 90, "dt_mom": 20.0, "dt_tracer": 20.0, "runlen": 22320.0, "AB_eps": 0.1, "x_origin": -149582.92913888372, "y_origin": 0.0, "pi": 3.141592653589793, "radius": 6370000.0, "degtom": 111177.4733520388, "omega": 7.292123516990375e-05, "rho_0": 1024.0, "grav": 9.81, "coord_degree": false, "enable_cyclic_x": false, "eq_of_state_type": 1, "enable_implicit_vert_friction": true, "enable_explicit_vert_friction": false, "enable_hor_friction": true, "enable_hor_diffusion": true, "enable_biharmonic_friction": false, "enable_biharmonic_mixing": false, "enable_hor_friction_cos_scaling": false, "enable_ray_friction": false, "enable_bottom_friction": false, "enable_bottom_friction_var": false, "enable_quadratic_bottom_friction": true, "enable_tempsalt_sources": false, "enable_momentum_sources": false, "enable_superbee_advection": false, "enable_conserve_energy": true, "enable_store_bottom_friction_tke": false, "enable_store_cabbeling_heat": false, "enable_noslip_lateral": false, "enable_streamfunction": false, "A_h": 10.0, "K_h": 10.0, "r_ray": 0.0, "r_bot": 0.0, "r_quad_bot": 0.001, "hor_friction_cosPower": 1.0, "A_hbi": 0.0, "K_hbi": 0.0, "biharmonic_friction_cosPower": 0.0, "kappaH_0": 0.0004, "kappaM_0": 0.0004, "enable_neutral_diffusion": false, "enable_skew_diffusion": false, "enable_TEM_friction": false, "K_iso_0": 0.0, "K_iso_steep": 0.0, "K_gm_0": 0.0, "iso_dslope": 0.0008, "iso_slopec": 0.001, "enable_idemix": false, "tau_v": 172800.0, "tau_h": 1296000.0, "gamma": 1.57, "jstar": 5.0, "mu0": 0.3333333333333333, "enable_idemix_hor_diffusion": false, "enable_eke_diss_bottom": false, "enable_eke_diss_surfbot": false, "eke_diss_surfbot_frac": 1.0, "enable_idemix_superbee_advection": false, "enable_idemix_upwind_advection": false, "enable_tke": false, "c_k": 0.1, "c_eps": 0.7, "alpha_tke": 1.0, "mxl_min": 1e-12, "kappaM_min": 0.0, "kappaM_max": 100.0, "tke_mxl_choice": 1, "enable_tke_superbee_advection": false, "enable_tke_upwind_advection": false, "enable_tke_hor_diffusion": false, "K_h_tke": 2000.0, "enable_eke": false, "eke_lmin": 100.0, "eke_c_k": 1.0, "eke_cross": 1.0, "eke_crhin": 1.0, "eke_c_eps": 1.0, "eke_k_max": 10000.0, "alpha_eke": 1.0, "enable_eke_superbee_advection": false, "enable_eke_upwind_advection": false, "enable_eke_isopycnal_diffusion": false, "restart_input_filename": null, "restart_output_filename": "{identifier}_{itt:0>4d}.restart.h5", "restart_frequency": 0.0, "kappaH_min": 0.0, "enable_kappaH_profile": false, "enable_Prandtl_tke": true, "Prandtl_tke0": 10.0}
- setup_file :
- /Users/jklymak/Dropbox/Teaching/Eos431Phy441/site/computing/example.py
- setup_code :
- #!/usr/bin/env python """ """ __VEROS_VERSION__ = '1.5.0' if __name__ == "__main__": raise RuntimeError( "Veros setups cannot be executed directly. " f"Try `veros run {__file__}` instead." ) # -- end of auto-generated header, original file below -- from veros import VerosSetup, veros_routine from veros.variables import allocate, Variable from veros.distributed import global_min, global_max, proc_index_to_rank, proc_rank_to_index from veros.core.operators import numpy as npx, update, at from veros.tools.setup import get_stretched_grid_steps from veros import runtime_settings as rs, runtime_state as rst class ChannelSetup(VerosSetup): """A model using cartesian coordinates in cyclic channel Wind forcing over a channel; no buoyancy """ @veros_routine def set_parameter(self, state): settings = state.settings settings.identifier = "example" settings.nx, settings.ny, settings.nz = 200, 4, 90 settings.dt_mom = 20 settings.dt_tracer = settings.dt_mom settings.runlen = 3600 * 6.2 settings.x_origin = 0.0 settings.y_origin = 0.0 settings.coord_degree = False settings.enable_cyclic_x = False settings.enable_neutral_diffusion = False settings.enable_hor_friction = True settings.enable_hor_diffusion = True settings.A_h = 10 settings.K_h = settings.A_h settings.enable_hor_friction_cos_scaling = False settings.hor_friction_cosPower = 1 settings.enable_quadratic_bottom_friction = True settings.r_quad_bot = 1e-3 settings.enable_implicit_vert_friction = True settings.enable_explicit_vert_friction = False settings.kappaM_0 = 4e-4 settings.kappaH_0 = 4e-4 settings.enable_streamfunction = False settings.enable_idemix = False settings.eq_of_state_type = 1 @veros_routine(dist_safe=False, local_variables=['dxt', 'dyt', 'dzt']) def set_grid(self, state): vs = state.variables settings = state.settings proc_idx = proc_rank_to_index(rst.proc_rank) print(proc_idx) ddz = 200 / settings.nz print(npx.shape(vs.dxt)) if False: vs.dxt = update(vs.dxt, at[...], 400) if True: for i in range(int(settings.nx / 3), -1, -1): vs.dxt = update(vs.dxt, at[i], vs.dxt[i+1] * 1.05) for i in range(int(2*settings.nx / 3)-1, settings.nx+4): vs.dxt = update(vs.dxt, at[i], vs.dxt[i-1] * 1.05) dx = get_stretched_grid_steps(102-25, 150e3, 400) vs.dxt = update(vs.dxt, at[...], npx.hstack((dx[::-1], npx.ones(50)*400, dx))) vs.dyt = update(vs.dyt, at[...], 1000) vs.dzt = update(vs.dzt, at[...], ddz) @veros_routine def set_coriolis(self, state): vs = state.variables # settings = state.settings vs.coriolis_t = update( vs.coriolis_t, at[...], 0e-4 ) @veros_routine(dist_safe=False, local_variables=['kbot', 'xt']) def set_topography(self, state): vs = state.variables # depth of deepest cell. 0 is land, 1 is deepest # nz is shallowest.. vs.kbot = update(vs.kbot, at[...], 1) kbot = npx.ones_like(vs.kbot[:, 0]) x = vs.xt x = x - npx.mean(x) kbot += npx.floor(35*npx.exp(-(x/25000)**2)).astype("int") for j in range(state.settings.ny+1): vs.kbot = update(vs.kbot, at[:, j], kbot) vs.kbot = update(vs.kbot, at[:, 0], 0) vs.kbot = update(vs.kbot, at[0, :], 0) @veros_routine(dist_safe=False, local_variables=['xu', 'xt', 'temp']) def set_initial_conditions(self, state): vs = state.variables settings = state.settings nx = settings.nx tbot = 10 ttop = 14 inx = npx.nonzero(vs.xt > npx.mean(vs.xt))[0] with settings.unlock(): settings.x_origin = -npx.mean(vs.xt) vs.xt = update(vs.xt, at[...], vs.xt+settings.x_origin) vs.xu = update(vs.xu, at[...], vs.xu+settings.x_origin) vs.temp = update(vs.temp, at[:inx[0], :, :, :], tbot) vs.temp = update(vs.temp, at[inx[0]:, :, :, :], ttop) vs.temp = update(vs.temp, at[:, :, :30, :], tbot) vs.temp = update(vs.temp, at[:, :, settings.nz-30:, :], ttop) @veros_routine def set_forcing(self, state): pass # vs = state.variables # vs.forc_temp_surface = vs.t_rest * (vs.t_star - vs.temp[:, :, -1, vs.tau]) @veros_routine def set_diagnostics(self, state): settings = state.settings diagnostics = state.diagnostics diagnostics["snapshot"].output_frequency = 7200 / 2 @veros_routine def after_timestep(self, state): pass
Compare to the full Dataset above, and note that yt
, yu
, and time
no longer have “Dimension”, which means the are just single valued in this new Dataset.
You can access variables in the Dataset using either a ds.temp
or ds['temp']
syntax. Note below that the temp
(temperature) variable just has two dimensions associated with it.
display(ds.temp)
<xarray.DataArray 'temp' (zt: 90, xt: 200)> [18000 values with dtype=float64] Coordinates: (5) Attributes: (2)
- zt: 90
- xt: 200
- ...
[18000 values with dtype=float64]
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- yt()float64-0.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array(-0.5)
- yu()float640.0
- long_name :
- Meridional coordinate (U)
- units :
- km
array(0.)
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- Time()timedelta64[ns]03:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array(10800000000000, dtype='timedelta64[ns]')
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
- long_name :
- Temperature
- units :
- deg C
You can also specify ranges of variables, so for instance the 3rd to 6th time values. Note that the new variables retain the Time
dimension.
with xr.open_dataset('example.snapshot.nc') as ds:
ds = ds.isel(yt=0, yu=0, Time=range(2,6))
display(ds)
display(ds.temp)
<xarray.Dataset> Dimensions: (xt: 200, xu: 200, zt: 90, zw: 90, tensor1: 2, tensor2: 2, Time: 4) Coordinates: (9) Data variables: (12/33) dxt (xt) float64 ... dxu (xu) float64 ... dyt float64 ... dyu float64 ... dzt (zt) float64 ... dzw (zw) float64 ... ... ... kappaM (Time, zt, xt) float64 ... kappaH (Time, zw, xt) float64 ... surface_taux (Time, xu) float64 ... surface_tauy (Time, xt) float64 ... forc_rho_surface (Time, xt) float64 ... psi (Time, xt) float64 ... Attributes: (7)
- xt: 200
- xu: 200
- zt: 90
- zw: 90
- tensor1: 2
- tensor2: 2
- Time: 4
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- xu(xu)float64-149.6 -146.1 ... 149.6 153.1
- long_name :
- Zonal coordinate (U)
- units :
- km
array([-1.495829e+02, -1.461175e+02, -1.426563e+02, -1.391999e+02, -1.357491e+02, -1.323045e+02, -1.288671e+02, -1.254377e+02, -1.220174e+02, -1.186076e+02, -1.152094e+02, -1.118246e+02, -1.084547e+02, -1.051018e+02, -1.017680e+02, -9.845565e+01, -9.516740e+01, -9.190616e+01, -8.867512e+01, -8.547776e+01, -8.231785e+01, -7.919946e+01, -7.612691e+01, -7.310480e+01, -7.013796e+01, -6.723138e+01, -6.439016e+01, -6.161946e+01, -5.892438e+01, -5.630987e+01, -5.378062e+01, -5.134095e+01, -4.899467e+01, -4.674498e+01, -4.459441e+01, -4.254466e+01, -4.059661e+01, -3.875025e+01, -3.700472e+01, -3.535830e+01, -3.380848e+01, -3.235205e+01, -3.098520e+01, -2.970360e+01, -2.850258e+01, -2.737717e+01, -2.632228e+01, -2.533277e+01, -2.440350e+01, -2.352950e+01, -2.270595e+01, -2.192824e+01, -2.119205e+01, -2.049330e+01, -1.982824e+01, -1.919337e+01, -1.858552e+01, -1.800177e+01, -1.743949e+01, -1.689630e+01, -1.637003e+01, -1.585878e+01, -1.536080e+01, -1.487457e+01, -1.439871e+01, -1.393201e+01, -1.347337e+01, -1.302184e+01, -1.257657e+01, -1.213681e+01, -1.170189e+01, -1.127124e+01, -1.084434e+01, -1.042072e+01, -1.000000e+01, -9.600000e+00, -9.200000e+00, -8.800000e+00, -8.400000e+00, -8.000000e+00, -7.600000e+00, -7.200000e+00, -6.800000e+00, -6.400000e+00, -6.000000e+00, -5.600000e+00, -5.200000e+00, -4.800000e+00, -4.400000e+00, -4.000000e+00, -3.600000e+00, -3.200000e+00, -2.800000e+00, -2.400000e+00, -2.000000e+00, -1.600000e+00, -1.200000e+00, -8.000000e-01, -4.000000e-01, 2.910383e-14, 4.000000e-01, 8.000000e-01, 1.200000e+00, 1.600000e+00, 2.000000e+00, 2.400000e+00, 2.800000e+00, 3.200000e+00, 3.600000e+00, 4.000000e+00, 4.400000e+00, 4.800000e+00, 5.200000e+00, 5.600000e+00, 6.000000e+00, 6.400000e+00, 6.800000e+00, 7.200000e+00, 7.600000e+00, 8.000000e+00, 8.400000e+00, 8.800000e+00, 9.200000e+00, 9.600000e+00, 1.000000e+01, 1.042072e+01, 1.084434e+01, 1.127124e+01, 1.170189e+01, 1.213681e+01, 1.257657e+01, 1.302184e+01, 1.347337e+01, 1.393201e+01, 1.439871e+01, 1.487457e+01, 1.536080e+01, 1.585878e+01, 1.637003e+01, 1.689630e+01, 1.743949e+01, 1.800177e+01, 1.858552e+01, 1.919337e+01, 1.982824e+01, 2.049330e+01, 2.119205e+01, 2.192824e+01, 2.270595e+01, 2.352950e+01, 2.440350e+01, 2.533277e+01, 2.632228e+01, 2.737717e+01, 2.850258e+01, 2.970360e+01, 3.098520e+01, 3.235205e+01, 3.380848e+01, 3.535830e+01, 3.700472e+01, 3.875025e+01, 4.059661e+01, 4.254466e+01, 4.459441e+01, 4.674498e+01, 4.899467e+01, 5.134095e+01, 5.378062e+01, 5.630987e+01, 5.892438e+01, 6.161946e+01, 6.439016e+01, 6.723138e+01, 7.013796e+01, 7.310480e+01, 7.612691e+01, 7.919946e+01, 8.231785e+01, 8.547776e+01, 8.867512e+01, 9.190616e+01, 9.516740e+01, 9.845565e+01, 1.017680e+02, 1.051018e+02, 1.084547e+02, 1.118246e+02, 1.152094e+02, 1.186076e+02, 1.220174e+02, 1.254377e+02, 1.288671e+02, 1.323045e+02, 1.357491e+02, 1.391999e+02, 1.426563e+02, 1.461175e+02, 1.495829e+02, 1.530521e+02])
- yt()float64-0.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array(-0.5)
- yu()float640.0
- long_name :
- Meridional coordinate (U)
- units :
- km
array(0.)
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- zw(zw)float64-197.8 -195.6 -193.3 ... -2.222 0.0
- long_name :
- Vertical coordinate (W)
- units :
- m
- positive :
- up
array([-197.777778, -195.555556, -193.333333, -191.111111, -188.888889, -186.666667, -184.444444, -182.222222, -180. , -177.777778, -175.555556, -173.333333, -171.111111, -168.888889, -166.666667, -164.444444, -162.222222, -160. , -157.777778, -155.555556, -153.333333, -151.111111, -148.888889, -146.666667, -144.444444, -142.222222, -140. , -137.777778, -135.555556, -133.333333, -131.111111, -128.888889, -126.666667, -124.444444, -122.222222, -120. , -117.777778, -115.555556, -113.333333, -111.111111, -108.888889, -106.666667, -104.444444, -102.222222, -100. , -97.777778, -95.555556, -93.333333, -91.111111, -88.888889, -86.666667, -84.444444, -82.222222, -80. , -77.777778, -75.555556, -73.333333, -71.111111, -68.888889, -66.666667, -64.444444, -62.222222, -60. , -57.777778, -55.555556, -53.333333, -51.111111, -48.888889, -46.666667, -44.444444, -42.222222, -40. , -37.777778, -35.555556, -33.333333, -31.111111, -28.888889, -26.666667, -24.444444, -22.222222, -20. , -17.777778, -15.555556, -13.333333, -11.111111, -8.888889, -6.666667, -4.444444, -2.222222, 0. ])
- tensor1(tensor1)float640.0 1.0
- long_name :
- tensor1
- units :
array([0., 1.])
- tensor2(tensor2)float640.0 1.0
- long_name :
- tensor2
- units :
array([0., 1.])
- Time(Time)timedelta64[ns]03:00:00 ... 06:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array([10800000000000, 14400000028800, 17999999971200, 21600000000000], dtype='timedelta64[ns]')
- dxt(xt)float64...
- long_name :
- Zonal T-grid spacing
- units :
- m
[200 values with dtype=float64]
- dxu(xu)float64...
- long_name :
- Zonal U-grid spacing
- units :
- m
[200 values with dtype=float64]
- dyt()float64...
- long_name :
- Meridional T-grid spacing
- units :
- m
[1 values with dtype=float64]
- dyu()float64...
- long_name :
- Meridional U-grid spacing
- units :
- m
[1 values with dtype=float64]
- dzt(zt)float64...
- long_name :
- Vertical spacing (T)
- units :
- m
[90 values with dtype=float64]
- dzw(zw)float64...
- long_name :
- Vertical spacing (W)
- units :
- m
[90 values with dtype=float64]
- ht(xt)float64...
- long_name :
- Total depth (T)
- units :
- m
[200 values with dtype=float64]
- hu(xu)float64...
- long_name :
- Total depth (U)
- units :
- m
[200 values with dtype=float64]
- hv(xt)float64...
- long_name :
- Total depth (V)
- units :
- m
[200 values with dtype=float64]
- beta(xt)float64...
- long_name :
- Change of Coriolis freq.
- units :
- 1/(ms)
[200 values with dtype=float64]
- area_t(xt)float64...
- long_name :
- Area of T-box
- units :
- m^2
[200 values with dtype=float64]
- area_u(xu)float64...
- long_name :
- Area of U-box
- units :
- m^2
[200 values with dtype=float64]
- area_v(xt)float64...
- long_name :
- Area of V-box
- units :
- m^2
[200 values with dtype=float64]
- rho(Time, zt, xt)float64...
- long_name :
- Density
- units :
- kg/m^3
[72000 values with dtype=float64]
- prho(Time, zt, xt)float64...
- long_name :
- Potential density
- units :
- kg/m^3
[72000 values with dtype=float64]
- int_drhodT(Time, zt, xt)float64...
- long_name :
- Der. of dyn. enthalpy by temperature
- units :
- kg / (m^2 deg C)
[72000 values with dtype=float64]
- int_drhodS(Time, zt, xt)float64...
- long_name :
- Der. of dyn. enthalpy by salinity
- units :
- kg / (m^2 g / kg)
[72000 values with dtype=float64]
- Nsqr(Time, zw, xt)float64...
- long_name :
- Square of stability frequency
- units :
- 1/s^2
[72000 values with dtype=float64]
- Hd(Time, zt, xt)float64...
- long_name :
- Dynamic enthalpy
- units :
- m^2/s^2
[72000 values with dtype=float64]
- temp(Time, zt, xt)float64...
- long_name :
- Temperature
- units :
- deg C
[72000 values with dtype=float64]
- salt(Time, zt, xt)float64...
- long_name :
- Salinity
- units :
- g/kg
[72000 values with dtype=float64]
- forc_temp_surface(Time, xt)float64...
- long_name :
- Surface temperature flux
- units :
- m deg C/s
[800 values with dtype=float64]
- forc_salt_surface(Time, xt)float64...
- long_name :
- Surface salinity flux
- units :
- m g/s kg
[800 values with dtype=float64]
- u(Time, zt, xu)float64...
- long_name :
- Zonal velocity
- units :
- m/s
[72000 values with dtype=float64]
- v(Time, zt, xt)float64...
- long_name :
- Meridional velocity
- units :
- m/s
[72000 values with dtype=float64]
- w(Time, zw, xt)float64...
- long_name :
- Vertical velocity
- units :
- m/s
[72000 values with dtype=float64]
- p_hydro(Time, zt, xt)float64...
- long_name :
- Hydrostatic pressure
- units :
- m^2/s^2
[72000 values with dtype=float64]
- kappaM(Time, zt, xt)float64...
- long_name :
- Vertical viscosity
- units :
- m^2/s
[72000 values with dtype=float64]
- kappaH(Time, zw, xt)float64...
- long_name :
- Vertical diffusivity
- units :
- m^2/s
[72000 values with dtype=float64]
- surface_taux(Time, xu)float64...
- long_name :
- Surface wind stress
- units :
- N/m^2
[800 values with dtype=float64]
- surface_tauy(Time, xt)float64...
- long_name :
- Surface wind stress
- units :
- N/m^2
[800 values with dtype=float64]
- forc_rho_surface(Time, xt)float64...
- long_name :
- Surface density flux
- units :
- kg / (m^2 s)
[800 values with dtype=float64]
- psi(Time, xt)float64...
- long_name :
- Surface pressure
- units :
- m^2/s^2
[800 values with dtype=float64]
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- xuPandasIndex
PandasIndex(Float64Index([ -149.5829291388837, -146.11747691514034, -142.656286565749, -139.19994298483698, -135.74910906034242, -132.3045354026015, -128.86707108785163, -125.43767546229275, -122.0174310337388, -118.60755745098363, ... 122.01743103373877, 125.43767546229269, 128.86707108785154, 132.3045354026014, 135.74910906034236, 139.19994298483692, 142.65628656574899, 146.11747691514034, 149.5829291388837, 153.05212756523898], dtype='float64', name='xu', length=200))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
- zwPandasIndex
PandasIndex(Float64Index([-197.77777777777814, -195.5555555555559, -193.33333333333368, -191.11111111111148, -188.88888888888926, -186.66666666666703, -184.4444444444448, -182.2222222222226, -180.00000000000037, -177.77777777777814, -175.5555555555559, -173.3333333333337, -171.11111111111148, -168.88888888888926, -166.66666666666703, -164.4444444444448, -162.2222222222226, -160.00000000000037, -157.77777777777814, -155.55555555555594, -153.3333333333337, -151.11111111111148, -148.88888888888926, -146.66666666666703, -144.44444444444483, -142.2222222222226, -140.00000000000037, -137.77777777777817, -135.55555555555594, -133.3333333333337, -131.11111111111148, -128.88888888888926, -126.66666666666703, -124.4444444444448, -122.22222222222257, -120.00000000000034, -117.77777777777811, -115.55555555555588, -113.33333333333366, -111.11111111111143, -108.8888888888892, -106.66666666666697, -104.44444444444474, -102.22222222222251, -100.00000000000028, -97.77777777777806, -95.55555555555583, -93.3333333333336, -91.11111111111137, -88.88888888888914, -86.66666666666691, -84.44444444444468, -82.22222222222246, -80.00000000000023, -77.777777777778, -75.55555555555577, -73.33333333333354, -71.11111111111131, -68.88888888888908, -66.66666666666686, -64.44444444444463, -62.2222222222224, -60.00000000000017, -57.77777777777794, -55.55555555555571, -53.333333333333485, -51.111111111111256, -48.88888888888903, -46.6666666666668, -44.44444444444457, -42.22222222222234, -40.000000000000114, -37.777777777777885, -35.55555555555566, -33.33333333333343, -31.1111111111112, -28.88888888888897, -26.666666666666742, -24.444444444444514, -22.222222222222285, -20.000000000000057, -17.77777777777783, -15.5555555555556, -13.333333333333371, -11.111111111111143, -8.888888888888914, -6.666666666666686, -4.444444444444457, -2.2222222222222285, 0.0], dtype='float64', name='zw'))
- tensor1PandasIndex
PandasIndex(Float64Index([0.0, 1.0], dtype='float64', name='tensor1'))
- tensor2PandasIndex
PandasIndex(Float64Index([0.0, 1.0], dtype='float64', name='tensor2'))
- TimePandasIndex
PandasIndex(TimedeltaIndex([ '0 days 03:00:00', '0 days 04:00:00.000028800', '0 days 04:59:59.999971200', '0 days 06:00:00'], dtype='timedelta64[ns]', name='Time', freq=None))
- date_created :
- 2023-09-07T15:00:26.245784
- veros_version :
- 1.5.0
- setup_identifier :
- example
- setup_description :
- setup_settings :
- {"identifier": "example", "description": "", "nx": 200, "ny": 4, "nz": 90, "dt_mom": 20.0, "dt_tracer": 20.0, "runlen": 22320.0, "AB_eps": 0.1, "x_origin": -149582.92913888372, "y_origin": 0.0, "pi": 3.141592653589793, "radius": 6370000.0, "degtom": 111177.4733520388, "omega": 7.292123516990375e-05, "rho_0": 1024.0, "grav": 9.81, "coord_degree": false, "enable_cyclic_x": false, "eq_of_state_type": 1, "enable_implicit_vert_friction": true, "enable_explicit_vert_friction": false, "enable_hor_friction": true, "enable_hor_diffusion": true, "enable_biharmonic_friction": false, "enable_biharmonic_mixing": false, "enable_hor_friction_cos_scaling": false, "enable_ray_friction": false, "enable_bottom_friction": false, "enable_bottom_friction_var": false, "enable_quadratic_bottom_friction": true, "enable_tempsalt_sources": false, "enable_momentum_sources": false, "enable_superbee_advection": false, "enable_conserve_energy": true, "enable_store_bottom_friction_tke": false, "enable_store_cabbeling_heat": false, "enable_noslip_lateral": false, "enable_streamfunction": false, "A_h": 10.0, "K_h": 10.0, "r_ray": 0.0, "r_bot": 0.0, "r_quad_bot": 0.001, "hor_friction_cosPower": 1.0, "A_hbi": 0.0, "K_hbi": 0.0, "biharmonic_friction_cosPower": 0.0, "kappaH_0": 0.0004, "kappaM_0": 0.0004, "enable_neutral_diffusion": false, "enable_skew_diffusion": false, "enable_TEM_friction": false, "K_iso_0": 0.0, "K_iso_steep": 0.0, "K_gm_0": 0.0, "iso_dslope": 0.0008, "iso_slopec": 0.001, "enable_idemix": false, "tau_v": 172800.0, "tau_h": 1296000.0, "gamma": 1.57, "jstar": 5.0, "mu0": 0.3333333333333333, "enable_idemix_hor_diffusion": false, "enable_eke_diss_bottom": false, "enable_eke_diss_surfbot": false, "eke_diss_surfbot_frac": 1.0, "enable_idemix_superbee_advection": false, "enable_idemix_upwind_advection": false, "enable_tke": false, "c_k": 0.1, "c_eps": 0.7, "alpha_tke": 1.0, "mxl_min": 1e-12, "kappaM_min": 0.0, "kappaM_max": 100.0, "tke_mxl_choice": 1, "enable_tke_superbee_advection": false, "enable_tke_upwind_advection": false, "enable_tke_hor_diffusion": false, "K_h_tke": 2000.0, "enable_eke": false, "eke_lmin": 100.0, "eke_c_k": 1.0, "eke_cross": 1.0, "eke_crhin": 1.0, "eke_c_eps": 1.0, "eke_k_max": 10000.0, "alpha_eke": 1.0, "enable_eke_superbee_advection": false, "enable_eke_upwind_advection": false, "enable_eke_isopycnal_diffusion": false, "restart_input_filename": null, "restart_output_filename": "{identifier}_{itt:0>4d}.restart.h5", "restart_frequency": 0.0, "kappaH_min": 0.0, "enable_kappaH_profile": false, "enable_Prandtl_tke": true, "Prandtl_tke0": 10.0}
- setup_file :
- /Users/jklymak/Dropbox/Teaching/Eos431Phy441/site/computing/example.py
- setup_code :
- #!/usr/bin/env python """ """ __VEROS_VERSION__ = '1.5.0' if __name__ == "__main__": raise RuntimeError( "Veros setups cannot be executed directly. " f"Try `veros run {__file__}` instead." ) # -- end of auto-generated header, original file below -- from veros import VerosSetup, veros_routine from veros.variables import allocate, Variable from veros.distributed import global_min, global_max, proc_index_to_rank, proc_rank_to_index from veros.core.operators import numpy as npx, update, at from veros.tools.setup import get_stretched_grid_steps from veros import runtime_settings as rs, runtime_state as rst class ChannelSetup(VerosSetup): """A model using cartesian coordinates in cyclic channel Wind forcing over a channel; no buoyancy """ @veros_routine def set_parameter(self, state): settings = state.settings settings.identifier = "example" settings.nx, settings.ny, settings.nz = 200, 4, 90 settings.dt_mom = 20 settings.dt_tracer = settings.dt_mom settings.runlen = 3600 * 6.2 settings.x_origin = 0.0 settings.y_origin = 0.0 settings.coord_degree = False settings.enable_cyclic_x = False settings.enable_neutral_diffusion = False settings.enable_hor_friction = True settings.enable_hor_diffusion = True settings.A_h = 10 settings.K_h = settings.A_h settings.enable_hor_friction_cos_scaling = False settings.hor_friction_cosPower = 1 settings.enable_quadratic_bottom_friction = True settings.r_quad_bot = 1e-3 settings.enable_implicit_vert_friction = True settings.enable_explicit_vert_friction = False settings.kappaM_0 = 4e-4 settings.kappaH_0 = 4e-4 settings.enable_streamfunction = False settings.enable_idemix = False settings.eq_of_state_type = 1 @veros_routine(dist_safe=False, local_variables=['dxt', 'dyt', 'dzt']) def set_grid(self, state): vs = state.variables settings = state.settings proc_idx = proc_rank_to_index(rst.proc_rank) print(proc_idx) ddz = 200 / settings.nz print(npx.shape(vs.dxt)) if False: vs.dxt = update(vs.dxt, at[...], 400) if True: for i in range(int(settings.nx / 3), -1, -1): vs.dxt = update(vs.dxt, at[i], vs.dxt[i+1] * 1.05) for i in range(int(2*settings.nx / 3)-1, settings.nx+4): vs.dxt = update(vs.dxt, at[i], vs.dxt[i-1] * 1.05) dx = get_stretched_grid_steps(102-25, 150e3, 400) vs.dxt = update(vs.dxt, at[...], npx.hstack((dx[::-1], npx.ones(50)*400, dx))) vs.dyt = update(vs.dyt, at[...], 1000) vs.dzt = update(vs.dzt, at[...], ddz) @veros_routine def set_coriolis(self, state): vs = state.variables # settings = state.settings vs.coriolis_t = update( vs.coriolis_t, at[...], 0e-4 ) @veros_routine(dist_safe=False, local_variables=['kbot', 'xt']) def set_topography(self, state): vs = state.variables # depth of deepest cell. 0 is land, 1 is deepest # nz is shallowest.. vs.kbot = update(vs.kbot, at[...], 1) kbot = npx.ones_like(vs.kbot[:, 0]) x = vs.xt x = x - npx.mean(x) kbot += npx.floor(35*npx.exp(-(x/25000)**2)).astype("int") for j in range(state.settings.ny+1): vs.kbot = update(vs.kbot, at[:, j], kbot) vs.kbot = update(vs.kbot, at[:, 0], 0) vs.kbot = update(vs.kbot, at[0, :], 0) @veros_routine(dist_safe=False, local_variables=['xu', 'xt', 'temp']) def set_initial_conditions(self, state): vs = state.variables settings = state.settings nx = settings.nx tbot = 10 ttop = 14 inx = npx.nonzero(vs.xt > npx.mean(vs.xt))[0] with settings.unlock(): settings.x_origin = -npx.mean(vs.xt) vs.xt = update(vs.xt, at[...], vs.xt+settings.x_origin) vs.xu = update(vs.xu, at[...], vs.xu+settings.x_origin) vs.temp = update(vs.temp, at[:inx[0], :, :, :], tbot) vs.temp = update(vs.temp, at[inx[0]:, :, :, :], ttop) vs.temp = update(vs.temp, at[:, :, :30, :], tbot) vs.temp = update(vs.temp, at[:, :, settings.nz-30:, :], ttop) @veros_routine def set_forcing(self, state): pass # vs = state.variables # vs.forc_temp_surface = vs.t_rest * (vs.t_star - vs.temp[:, :, -1, vs.tau]) @veros_routine def set_diagnostics(self, state): settings = state.settings diagnostics = state.diagnostics diagnostics["snapshot"].output_frequency = 7200 / 2 @veros_routine def after_timestep(self, state): pass
<xarray.DataArray 'temp' (Time: 4, zt: 90, xt: 200)> [72000 values with dtype=float64] Coordinates: (5) Attributes: (2)
- Time: 4
- zt: 90
- xt: 200
- ...
[72000 values with dtype=float64]
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- yt()float64-0.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array(-0.5)
- yu()float640.0
- long_name :
- Meridional coordinate (U)
- units :
- km
array(0.)
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- Time(Time)timedelta64[ns]03:00:00 ... 06:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array([10800000000000, 14400000028800, 17999999971200, 21600000000000], dtype='timedelta64[ns]')
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
- TimePandasIndex
PandasIndex(TimedeltaIndex([ '0 days 03:00:00', '0 days 04:00:00.000028800', '0 days 04:59:59.999971200', '0 days 06:00:00'], dtype='timedelta64[ns]', name='Time', freq=None))
- long_name :
- Temperature
- units :
- deg C
Selecting data by coordinate value: sel
#
If you want to work with values of coordinates, rather than indices, that is possible using the sel
method. Note here we use slice
notation to specify a range in time. np.timedelta64(6, 'h')
means 6 hours from the start of the simulation.
with xr.open_dataset('example.snapshot.nc') as ds:
# get data between 6 and 11 hourse since start of simulation:
ds = ds.isel(yt=0, yu=0).sel(Time=slice(np.timedelta64(6, 'h'), np.timedelta64(11, 'h')))
display(ds)
print(ds.Time.values)
<xarray.Dataset> Dimensions: (xt: 200, xu: 200, zt: 90, zw: 90, tensor1: 2, tensor2: 2, Time: 1) Coordinates: (9) Data variables: (12/33) dxt (xt) float64 ... dxu (xu) float64 ... dyt float64 ... dyu float64 ... dzt (zt) float64 ... dzw (zw) float64 ... ... ... kappaM (Time, zt, xt) float64 ... kappaH (Time, zw, xt) float64 ... surface_taux (Time, xu) float64 ... surface_tauy (Time, xt) float64 ... forc_rho_surface (Time, xt) float64 ... psi (Time, xt) float64 ... Attributes: (7)
- xt: 200
- xu: 200
- zt: 90
- zw: 90
- tensor1: 2
- tensor2: 2
- Time: 1
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- xu(xu)float64-149.6 -146.1 ... 149.6 153.1
- long_name :
- Zonal coordinate (U)
- units :
- km
array([-1.495829e+02, -1.461175e+02, -1.426563e+02, -1.391999e+02, -1.357491e+02, -1.323045e+02, -1.288671e+02, -1.254377e+02, -1.220174e+02, -1.186076e+02, -1.152094e+02, -1.118246e+02, -1.084547e+02, -1.051018e+02, -1.017680e+02, -9.845565e+01, -9.516740e+01, -9.190616e+01, -8.867512e+01, -8.547776e+01, -8.231785e+01, -7.919946e+01, -7.612691e+01, -7.310480e+01, -7.013796e+01, -6.723138e+01, -6.439016e+01, -6.161946e+01, -5.892438e+01, -5.630987e+01, -5.378062e+01, -5.134095e+01, -4.899467e+01, -4.674498e+01, -4.459441e+01, -4.254466e+01, -4.059661e+01, -3.875025e+01, -3.700472e+01, -3.535830e+01, -3.380848e+01, -3.235205e+01, -3.098520e+01, -2.970360e+01, -2.850258e+01, -2.737717e+01, -2.632228e+01, -2.533277e+01, -2.440350e+01, -2.352950e+01, -2.270595e+01, -2.192824e+01, -2.119205e+01, -2.049330e+01, -1.982824e+01, -1.919337e+01, -1.858552e+01, -1.800177e+01, -1.743949e+01, -1.689630e+01, -1.637003e+01, -1.585878e+01, -1.536080e+01, -1.487457e+01, -1.439871e+01, -1.393201e+01, -1.347337e+01, -1.302184e+01, -1.257657e+01, -1.213681e+01, -1.170189e+01, -1.127124e+01, -1.084434e+01, -1.042072e+01, -1.000000e+01, -9.600000e+00, -9.200000e+00, -8.800000e+00, -8.400000e+00, -8.000000e+00, -7.600000e+00, -7.200000e+00, -6.800000e+00, -6.400000e+00, -6.000000e+00, -5.600000e+00, -5.200000e+00, -4.800000e+00, -4.400000e+00, -4.000000e+00, -3.600000e+00, -3.200000e+00, -2.800000e+00, -2.400000e+00, -2.000000e+00, -1.600000e+00, -1.200000e+00, -8.000000e-01, -4.000000e-01, 2.910383e-14, 4.000000e-01, 8.000000e-01, 1.200000e+00, 1.600000e+00, 2.000000e+00, 2.400000e+00, 2.800000e+00, 3.200000e+00, 3.600000e+00, 4.000000e+00, 4.400000e+00, 4.800000e+00, 5.200000e+00, 5.600000e+00, 6.000000e+00, 6.400000e+00, 6.800000e+00, 7.200000e+00, 7.600000e+00, 8.000000e+00, 8.400000e+00, 8.800000e+00, 9.200000e+00, 9.600000e+00, 1.000000e+01, 1.042072e+01, 1.084434e+01, 1.127124e+01, 1.170189e+01, 1.213681e+01, 1.257657e+01, 1.302184e+01, 1.347337e+01, 1.393201e+01, 1.439871e+01, 1.487457e+01, 1.536080e+01, 1.585878e+01, 1.637003e+01, 1.689630e+01, 1.743949e+01, 1.800177e+01, 1.858552e+01, 1.919337e+01, 1.982824e+01, 2.049330e+01, 2.119205e+01, 2.192824e+01, 2.270595e+01, 2.352950e+01, 2.440350e+01, 2.533277e+01, 2.632228e+01, 2.737717e+01, 2.850258e+01, 2.970360e+01, 3.098520e+01, 3.235205e+01, 3.380848e+01, 3.535830e+01, 3.700472e+01, 3.875025e+01, 4.059661e+01, 4.254466e+01, 4.459441e+01, 4.674498e+01, 4.899467e+01, 5.134095e+01, 5.378062e+01, 5.630987e+01, 5.892438e+01, 6.161946e+01, 6.439016e+01, 6.723138e+01, 7.013796e+01, 7.310480e+01, 7.612691e+01, 7.919946e+01, 8.231785e+01, 8.547776e+01, 8.867512e+01, 9.190616e+01, 9.516740e+01, 9.845565e+01, 1.017680e+02, 1.051018e+02, 1.084547e+02, 1.118246e+02, 1.152094e+02, 1.186076e+02, 1.220174e+02, 1.254377e+02, 1.288671e+02, 1.323045e+02, 1.357491e+02, 1.391999e+02, 1.426563e+02, 1.461175e+02, 1.495829e+02, 1.530521e+02])
- yt()float64-0.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array(-0.5)
- yu()float640.0
- long_name :
- Meridional coordinate (U)
- units :
- km
array(0.)
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- zw(zw)float64-197.8 -195.6 -193.3 ... -2.222 0.0
- long_name :
- Vertical coordinate (W)
- units :
- m
- positive :
- up
array([-197.777778, -195.555556, -193.333333, -191.111111, -188.888889, -186.666667, -184.444444, -182.222222, -180. , -177.777778, -175.555556, -173.333333, -171.111111, -168.888889, -166.666667, -164.444444, -162.222222, -160. , -157.777778, -155.555556, -153.333333, -151.111111, -148.888889, -146.666667, -144.444444, -142.222222, -140. , -137.777778, -135.555556, -133.333333, -131.111111, -128.888889, -126.666667, -124.444444, -122.222222, -120. , -117.777778, -115.555556, -113.333333, -111.111111, -108.888889, -106.666667, -104.444444, -102.222222, -100. , -97.777778, -95.555556, -93.333333, -91.111111, -88.888889, -86.666667, -84.444444, -82.222222, -80. , -77.777778, -75.555556, -73.333333, -71.111111, -68.888889, -66.666667, -64.444444, -62.222222, -60. , -57.777778, -55.555556, -53.333333, -51.111111, -48.888889, -46.666667, -44.444444, -42.222222, -40. , -37.777778, -35.555556, -33.333333, -31.111111, -28.888889, -26.666667, -24.444444, -22.222222, -20. , -17.777778, -15.555556, -13.333333, -11.111111, -8.888889, -6.666667, -4.444444, -2.222222, 0. ])
- tensor1(tensor1)float640.0 1.0
- long_name :
- tensor1
- units :
array([0., 1.])
- tensor2(tensor2)float640.0 1.0
- long_name :
- tensor2
- units :
array([0., 1.])
- Time(Time)timedelta64[ns]06:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array([21600000000000], dtype='timedelta64[ns]')
- dxt(xt)float64...
- long_name :
- Zonal T-grid spacing
- units :
- m
[200 values with dtype=float64]
- dxu(xu)float64...
- long_name :
- Zonal U-grid spacing
- units :
- m
[200 values with dtype=float64]
- dyt()float64...
- long_name :
- Meridional T-grid spacing
- units :
- m
[1 values with dtype=float64]
- dyu()float64...
- long_name :
- Meridional U-grid spacing
- units :
- m
[1 values with dtype=float64]
- dzt(zt)float64...
- long_name :
- Vertical spacing (T)
- units :
- m
[90 values with dtype=float64]
- dzw(zw)float64...
- long_name :
- Vertical spacing (W)
- units :
- m
[90 values with dtype=float64]
- ht(xt)float64...
- long_name :
- Total depth (T)
- units :
- m
[200 values with dtype=float64]
- hu(xu)float64...
- long_name :
- Total depth (U)
- units :
- m
[200 values with dtype=float64]
- hv(xt)float64...
- long_name :
- Total depth (V)
- units :
- m
[200 values with dtype=float64]
- beta(xt)float64...
- long_name :
- Change of Coriolis freq.
- units :
- 1/(ms)
[200 values with dtype=float64]
- area_t(xt)float64...
- long_name :
- Area of T-box
- units :
- m^2
[200 values with dtype=float64]
- area_u(xu)float64...
- long_name :
- Area of U-box
- units :
- m^2
[200 values with dtype=float64]
- area_v(xt)float64...
- long_name :
- Area of V-box
- units :
- m^2
[200 values with dtype=float64]
- rho(Time, zt, xt)float64...
- long_name :
- Density
- units :
- kg/m^3
[18000 values with dtype=float64]
- prho(Time, zt, xt)float64...
- long_name :
- Potential density
- units :
- kg/m^3
[18000 values with dtype=float64]
- int_drhodT(Time, zt, xt)float64...
- long_name :
- Der. of dyn. enthalpy by temperature
- units :
- kg / (m^2 deg C)
[18000 values with dtype=float64]
- int_drhodS(Time, zt, xt)float64...
- long_name :
- Der. of dyn. enthalpy by salinity
- units :
- kg / (m^2 g / kg)
[18000 values with dtype=float64]
- Nsqr(Time, zw, xt)float64...
- long_name :
- Square of stability frequency
- units :
- 1/s^2
[18000 values with dtype=float64]
- Hd(Time, zt, xt)float64...
- long_name :
- Dynamic enthalpy
- units :
- m^2/s^2
[18000 values with dtype=float64]
- temp(Time, zt, xt)float64...
- long_name :
- Temperature
- units :
- deg C
[18000 values with dtype=float64]
- salt(Time, zt, xt)float64...
- long_name :
- Salinity
- units :
- g/kg
[18000 values with dtype=float64]
- forc_temp_surface(Time, xt)float64...
- long_name :
- Surface temperature flux
- units :
- m deg C/s
[200 values with dtype=float64]
- forc_salt_surface(Time, xt)float64...
- long_name :
- Surface salinity flux
- units :
- m g/s kg
[200 values with dtype=float64]
- u(Time, zt, xu)float64...
- long_name :
- Zonal velocity
- units :
- m/s
[18000 values with dtype=float64]
- v(Time, zt, xt)float64...
- long_name :
- Meridional velocity
- units :
- m/s
[18000 values with dtype=float64]
- w(Time, zw, xt)float64...
- long_name :
- Vertical velocity
- units :
- m/s
[18000 values with dtype=float64]
- p_hydro(Time, zt, xt)float64...
- long_name :
- Hydrostatic pressure
- units :
- m^2/s^2
[18000 values with dtype=float64]
- kappaM(Time, zt, xt)float64...
- long_name :
- Vertical viscosity
- units :
- m^2/s
[18000 values with dtype=float64]
- kappaH(Time, zw, xt)float64...
- long_name :
- Vertical diffusivity
- units :
- m^2/s
[18000 values with dtype=float64]
- surface_taux(Time, xu)float64...
- long_name :
- Surface wind stress
- units :
- N/m^2
[200 values with dtype=float64]
- surface_tauy(Time, xt)float64...
- long_name :
- Surface wind stress
- units :
- N/m^2
[200 values with dtype=float64]
- forc_rho_surface(Time, xt)float64...
- long_name :
- Surface density flux
- units :
- kg / (m^2 s)
[200 values with dtype=float64]
- psi(Time, xt)float64...
- long_name :
- Surface pressure
- units :
- m^2/s^2
[200 values with dtype=float64]
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- xuPandasIndex
PandasIndex(Float64Index([ -149.5829291388837, -146.11747691514034, -142.656286565749, -139.19994298483698, -135.74910906034242, -132.3045354026015, -128.86707108785163, -125.43767546229275, -122.0174310337388, -118.60755745098363, ... 122.01743103373877, 125.43767546229269, 128.86707108785154, 132.3045354026014, 135.74910906034236, 139.19994298483692, 142.65628656574899, 146.11747691514034, 149.5829291388837, 153.05212756523898], dtype='float64', name='xu', length=200))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
- zwPandasIndex
PandasIndex(Float64Index([-197.77777777777814, -195.5555555555559, -193.33333333333368, -191.11111111111148, -188.88888888888926, -186.66666666666703, -184.4444444444448, -182.2222222222226, -180.00000000000037, -177.77777777777814, -175.5555555555559, -173.3333333333337, -171.11111111111148, -168.88888888888926, -166.66666666666703, -164.4444444444448, -162.2222222222226, -160.00000000000037, -157.77777777777814, -155.55555555555594, -153.3333333333337, -151.11111111111148, -148.88888888888926, -146.66666666666703, -144.44444444444483, -142.2222222222226, -140.00000000000037, -137.77777777777817, -135.55555555555594, -133.3333333333337, -131.11111111111148, -128.88888888888926, -126.66666666666703, -124.4444444444448, -122.22222222222257, -120.00000000000034, -117.77777777777811, -115.55555555555588, -113.33333333333366, -111.11111111111143, -108.8888888888892, -106.66666666666697, -104.44444444444474, -102.22222222222251, -100.00000000000028, -97.77777777777806, -95.55555555555583, -93.3333333333336, -91.11111111111137, -88.88888888888914, -86.66666666666691, -84.44444444444468, -82.22222222222246, -80.00000000000023, -77.777777777778, -75.55555555555577, -73.33333333333354, -71.11111111111131, -68.88888888888908, -66.66666666666686, -64.44444444444463, -62.2222222222224, -60.00000000000017, -57.77777777777794, -55.55555555555571, -53.333333333333485, -51.111111111111256, -48.88888888888903, -46.6666666666668, -44.44444444444457, -42.22222222222234, -40.000000000000114, -37.777777777777885, -35.55555555555566, -33.33333333333343, -31.1111111111112, -28.88888888888897, -26.666666666666742, -24.444444444444514, -22.222222222222285, -20.000000000000057, -17.77777777777783, -15.5555555555556, -13.333333333333371, -11.111111111111143, -8.888888888888914, -6.666666666666686, -4.444444444444457, -2.2222222222222285, 0.0], dtype='float64', name='zw'))
- tensor1PandasIndex
PandasIndex(Float64Index([0.0, 1.0], dtype='float64', name='tensor1'))
- tensor2PandasIndex
PandasIndex(Float64Index([0.0, 1.0], dtype='float64', name='tensor2'))
- TimePandasIndex
PandasIndex(TimedeltaIndex(['0 days 06:00:00'], dtype='timedelta64[ns]', name='Time', freq=None))
- date_created :
- 2023-09-07T15:00:26.245784
- veros_version :
- 1.5.0
- setup_identifier :
- example
- setup_description :
- setup_settings :
- {"identifier": "example", "description": "", "nx": 200, "ny": 4, "nz": 90, "dt_mom": 20.0, "dt_tracer": 20.0, "runlen": 22320.0, "AB_eps": 0.1, "x_origin": -149582.92913888372, "y_origin": 0.0, "pi": 3.141592653589793, "radius": 6370000.0, "degtom": 111177.4733520388, "omega": 7.292123516990375e-05, "rho_0": 1024.0, "grav": 9.81, "coord_degree": false, "enable_cyclic_x": false, "eq_of_state_type": 1, "enable_implicit_vert_friction": true, "enable_explicit_vert_friction": false, "enable_hor_friction": true, "enable_hor_diffusion": true, "enable_biharmonic_friction": false, "enable_biharmonic_mixing": false, "enable_hor_friction_cos_scaling": false, "enable_ray_friction": false, "enable_bottom_friction": false, "enable_bottom_friction_var": false, "enable_quadratic_bottom_friction": true, "enable_tempsalt_sources": false, "enable_momentum_sources": false, "enable_superbee_advection": false, "enable_conserve_energy": true, "enable_store_bottom_friction_tke": false, "enable_store_cabbeling_heat": false, "enable_noslip_lateral": false, "enable_streamfunction": false, "A_h": 10.0, "K_h": 10.0, "r_ray": 0.0, "r_bot": 0.0, "r_quad_bot": 0.001, "hor_friction_cosPower": 1.0, "A_hbi": 0.0, "K_hbi": 0.0, "biharmonic_friction_cosPower": 0.0, "kappaH_0": 0.0004, "kappaM_0": 0.0004, "enable_neutral_diffusion": false, "enable_skew_diffusion": false, "enable_TEM_friction": false, "K_iso_0": 0.0, "K_iso_steep": 0.0, "K_gm_0": 0.0, "iso_dslope": 0.0008, "iso_slopec": 0.001, "enable_idemix": false, "tau_v": 172800.0, "tau_h": 1296000.0, "gamma": 1.57, "jstar": 5.0, "mu0": 0.3333333333333333, "enable_idemix_hor_diffusion": false, "enable_eke_diss_bottom": false, "enable_eke_diss_surfbot": false, "eke_diss_surfbot_frac": 1.0, "enable_idemix_superbee_advection": false, "enable_idemix_upwind_advection": false, "enable_tke": false, "c_k": 0.1, "c_eps": 0.7, "alpha_tke": 1.0, "mxl_min": 1e-12, "kappaM_min": 0.0, "kappaM_max": 100.0, "tke_mxl_choice": 1, "enable_tke_superbee_advection": false, "enable_tke_upwind_advection": false, "enable_tke_hor_diffusion": false, "K_h_tke": 2000.0, "enable_eke": false, "eke_lmin": 100.0, "eke_c_k": 1.0, "eke_cross": 1.0, "eke_crhin": 1.0, "eke_c_eps": 1.0, "eke_k_max": 10000.0, "alpha_eke": 1.0, "enable_eke_superbee_advection": false, "enable_eke_upwind_advection": false, "enable_eke_isopycnal_diffusion": false, "restart_input_filename": null, "restart_output_filename": "{identifier}_{itt:0>4d}.restart.h5", "restart_frequency": 0.0, "kappaH_min": 0.0, "enable_kappaH_profile": false, "enable_Prandtl_tke": true, "Prandtl_tke0": 10.0}
- setup_file :
- /Users/jklymak/Dropbox/Teaching/Eos431Phy441/site/computing/example.py
- setup_code :
- #!/usr/bin/env python """ """ __VEROS_VERSION__ = '1.5.0' if __name__ == "__main__": raise RuntimeError( "Veros setups cannot be executed directly. " f"Try `veros run {__file__}` instead." ) # -- end of auto-generated header, original file below -- from veros import VerosSetup, veros_routine from veros.variables import allocate, Variable from veros.distributed import global_min, global_max, proc_index_to_rank, proc_rank_to_index from veros.core.operators import numpy as npx, update, at from veros.tools.setup import get_stretched_grid_steps from veros import runtime_settings as rs, runtime_state as rst class ChannelSetup(VerosSetup): """A model using cartesian coordinates in cyclic channel Wind forcing over a channel; no buoyancy """ @veros_routine def set_parameter(self, state): settings = state.settings settings.identifier = "example" settings.nx, settings.ny, settings.nz = 200, 4, 90 settings.dt_mom = 20 settings.dt_tracer = settings.dt_mom settings.runlen = 3600 * 6.2 settings.x_origin = 0.0 settings.y_origin = 0.0 settings.coord_degree = False settings.enable_cyclic_x = False settings.enable_neutral_diffusion = False settings.enable_hor_friction = True settings.enable_hor_diffusion = True settings.A_h = 10 settings.K_h = settings.A_h settings.enable_hor_friction_cos_scaling = False settings.hor_friction_cosPower = 1 settings.enable_quadratic_bottom_friction = True settings.r_quad_bot = 1e-3 settings.enable_implicit_vert_friction = True settings.enable_explicit_vert_friction = False settings.kappaM_0 = 4e-4 settings.kappaH_0 = 4e-4 settings.enable_streamfunction = False settings.enable_idemix = False settings.eq_of_state_type = 1 @veros_routine(dist_safe=False, local_variables=['dxt', 'dyt', 'dzt']) def set_grid(self, state): vs = state.variables settings = state.settings proc_idx = proc_rank_to_index(rst.proc_rank) print(proc_idx) ddz = 200 / settings.nz print(npx.shape(vs.dxt)) if False: vs.dxt = update(vs.dxt, at[...], 400) if True: for i in range(int(settings.nx / 3), -1, -1): vs.dxt = update(vs.dxt, at[i], vs.dxt[i+1] * 1.05) for i in range(int(2*settings.nx / 3)-1, settings.nx+4): vs.dxt = update(vs.dxt, at[i], vs.dxt[i-1] * 1.05) dx = get_stretched_grid_steps(102-25, 150e3, 400) vs.dxt = update(vs.dxt, at[...], npx.hstack((dx[::-1], npx.ones(50)*400, dx))) vs.dyt = update(vs.dyt, at[...], 1000) vs.dzt = update(vs.dzt, at[...], ddz) @veros_routine def set_coriolis(self, state): vs = state.variables # settings = state.settings vs.coriolis_t = update( vs.coriolis_t, at[...], 0e-4 ) @veros_routine(dist_safe=False, local_variables=['kbot', 'xt']) def set_topography(self, state): vs = state.variables # depth of deepest cell. 0 is land, 1 is deepest # nz is shallowest.. vs.kbot = update(vs.kbot, at[...], 1) kbot = npx.ones_like(vs.kbot[:, 0]) x = vs.xt x = x - npx.mean(x) kbot += npx.floor(35*npx.exp(-(x/25000)**2)).astype("int") for j in range(state.settings.ny+1): vs.kbot = update(vs.kbot, at[:, j], kbot) vs.kbot = update(vs.kbot, at[:, 0], 0) vs.kbot = update(vs.kbot, at[0, :], 0) @veros_routine(dist_safe=False, local_variables=['xu', 'xt', 'temp']) def set_initial_conditions(self, state): vs = state.variables settings = state.settings nx = settings.nx tbot = 10 ttop = 14 inx = npx.nonzero(vs.xt > npx.mean(vs.xt))[0] with settings.unlock(): settings.x_origin = -npx.mean(vs.xt) vs.xt = update(vs.xt, at[...], vs.xt+settings.x_origin) vs.xu = update(vs.xu, at[...], vs.xu+settings.x_origin) vs.temp = update(vs.temp, at[:inx[0], :, :, :], tbot) vs.temp = update(vs.temp, at[inx[0]:, :, :, :], ttop) vs.temp = update(vs.temp, at[:, :, :30, :], tbot) vs.temp = update(vs.temp, at[:, :, settings.nz-30:, :], ttop) @veros_routine def set_forcing(self, state): pass # vs = state.variables # vs.forc_temp_surface = vs.t_rest * (vs.t_star - vs.temp[:, :, -1, vs.tau]) @veros_routine def set_diagnostics(self, state): settings = state.settings diagnostics = state.diagnostics diagnostics["snapshot"].output_frequency = 7200 / 2 @veros_routine def after_timestep(self, state): pass
[21600000000000]
If we would prefer to just get one time value then that is easy if we choose a co-ordinate value:
with xr.open_dataset('example.snapshot.nc') as ds:
ds = ds.isel(yt=0, yu=0).sel(Time=np.timedelta64(6, 'h'))
display(ds)
<xarray.Dataset> Dimensions: (xt: 200, xu: 200, zt: 90, zw: 90, tensor1: 2, tensor2: 2) Coordinates: (9) Data variables: (12/33) dxt (xt) float64 ... dxu (xu) float64 ... dyt float64 ... dyu float64 ... dzt (zt) float64 ... dzw (zw) float64 ... ... ... kappaM (zt, xt) float64 ... kappaH (zw, xt) float64 ... surface_taux (xu) float64 ... surface_tauy (xt) float64 ... forc_rho_surface (xt) float64 ... psi (xt) float64 ... Attributes: (7)
- xt: 200
- xu: 200
- zt: 90
- zw: 90
- tensor1: 2
- tensor2: 2
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- xu(xu)float64-149.6 -146.1 ... 149.6 153.1
- long_name :
- Zonal coordinate (U)
- units :
- km
array([-1.495829e+02, -1.461175e+02, -1.426563e+02, -1.391999e+02, -1.357491e+02, -1.323045e+02, -1.288671e+02, -1.254377e+02, -1.220174e+02, -1.186076e+02, -1.152094e+02, -1.118246e+02, -1.084547e+02, -1.051018e+02, -1.017680e+02, -9.845565e+01, -9.516740e+01, -9.190616e+01, -8.867512e+01, -8.547776e+01, -8.231785e+01, -7.919946e+01, -7.612691e+01, -7.310480e+01, -7.013796e+01, -6.723138e+01, -6.439016e+01, -6.161946e+01, -5.892438e+01, -5.630987e+01, -5.378062e+01, -5.134095e+01, -4.899467e+01, -4.674498e+01, -4.459441e+01, -4.254466e+01, -4.059661e+01, -3.875025e+01, -3.700472e+01, -3.535830e+01, -3.380848e+01, -3.235205e+01, -3.098520e+01, -2.970360e+01, -2.850258e+01, -2.737717e+01, -2.632228e+01, -2.533277e+01, -2.440350e+01, -2.352950e+01, -2.270595e+01, -2.192824e+01, -2.119205e+01, -2.049330e+01, -1.982824e+01, -1.919337e+01, -1.858552e+01, -1.800177e+01, -1.743949e+01, -1.689630e+01, -1.637003e+01, -1.585878e+01, -1.536080e+01, -1.487457e+01, -1.439871e+01, -1.393201e+01, -1.347337e+01, -1.302184e+01, -1.257657e+01, -1.213681e+01, -1.170189e+01, -1.127124e+01, -1.084434e+01, -1.042072e+01, -1.000000e+01, -9.600000e+00, -9.200000e+00, -8.800000e+00, -8.400000e+00, -8.000000e+00, -7.600000e+00, -7.200000e+00, -6.800000e+00, -6.400000e+00, -6.000000e+00, -5.600000e+00, -5.200000e+00, -4.800000e+00, -4.400000e+00, -4.000000e+00, -3.600000e+00, -3.200000e+00, -2.800000e+00, -2.400000e+00, -2.000000e+00, -1.600000e+00, -1.200000e+00, -8.000000e-01, -4.000000e-01, 2.910383e-14, 4.000000e-01, 8.000000e-01, 1.200000e+00, 1.600000e+00, 2.000000e+00, 2.400000e+00, 2.800000e+00, 3.200000e+00, 3.600000e+00, 4.000000e+00, 4.400000e+00, 4.800000e+00, 5.200000e+00, 5.600000e+00, 6.000000e+00, 6.400000e+00, 6.800000e+00, 7.200000e+00, 7.600000e+00, 8.000000e+00, 8.400000e+00, 8.800000e+00, 9.200000e+00, 9.600000e+00, 1.000000e+01, 1.042072e+01, 1.084434e+01, 1.127124e+01, 1.170189e+01, 1.213681e+01, 1.257657e+01, 1.302184e+01, 1.347337e+01, 1.393201e+01, 1.439871e+01, 1.487457e+01, 1.536080e+01, 1.585878e+01, 1.637003e+01, 1.689630e+01, 1.743949e+01, 1.800177e+01, 1.858552e+01, 1.919337e+01, 1.982824e+01, 2.049330e+01, 2.119205e+01, 2.192824e+01, 2.270595e+01, 2.352950e+01, 2.440350e+01, 2.533277e+01, 2.632228e+01, 2.737717e+01, 2.850258e+01, 2.970360e+01, 3.098520e+01, 3.235205e+01, 3.380848e+01, 3.535830e+01, 3.700472e+01, 3.875025e+01, 4.059661e+01, 4.254466e+01, 4.459441e+01, 4.674498e+01, 4.899467e+01, 5.134095e+01, 5.378062e+01, 5.630987e+01, 5.892438e+01, 6.161946e+01, 6.439016e+01, 6.723138e+01, 7.013796e+01, 7.310480e+01, 7.612691e+01, 7.919946e+01, 8.231785e+01, 8.547776e+01, 8.867512e+01, 9.190616e+01, 9.516740e+01, 9.845565e+01, 1.017680e+02, 1.051018e+02, 1.084547e+02, 1.118246e+02, 1.152094e+02, 1.186076e+02, 1.220174e+02, 1.254377e+02, 1.288671e+02, 1.323045e+02, 1.357491e+02, 1.391999e+02, 1.426563e+02, 1.461175e+02, 1.495829e+02, 1.530521e+02])
- yt()float64-0.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array(-0.5)
- yu()float640.0
- long_name :
- Meridional coordinate (U)
- units :
- km
array(0.)
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- zw(zw)float64-197.8 -195.6 -193.3 ... -2.222 0.0
- long_name :
- Vertical coordinate (W)
- units :
- m
- positive :
- up
array([-197.777778, -195.555556, -193.333333, -191.111111, -188.888889, -186.666667, -184.444444, -182.222222, -180. , -177.777778, -175.555556, -173.333333, -171.111111, -168.888889, -166.666667, -164.444444, -162.222222, -160. , -157.777778, -155.555556, -153.333333, -151.111111, -148.888889, -146.666667, -144.444444, -142.222222, -140. , -137.777778, -135.555556, -133.333333, -131.111111, -128.888889, -126.666667, -124.444444, -122.222222, -120. , -117.777778, -115.555556, -113.333333, -111.111111, -108.888889, -106.666667, -104.444444, -102.222222, -100. , -97.777778, -95.555556, -93.333333, -91.111111, -88.888889, -86.666667, -84.444444, -82.222222, -80. , -77.777778, -75.555556, -73.333333, -71.111111, -68.888889, -66.666667, -64.444444, -62.222222, -60. , -57.777778, -55.555556, -53.333333, -51.111111, -48.888889, -46.666667, -44.444444, -42.222222, -40. , -37.777778, -35.555556, -33.333333, -31.111111, -28.888889, -26.666667, -24.444444, -22.222222, -20. , -17.777778, -15.555556, -13.333333, -11.111111, -8.888889, -6.666667, -4.444444, -2.222222, 0. ])
- tensor1(tensor1)float640.0 1.0
- long_name :
- tensor1
- units :
array([0., 1.])
- tensor2(tensor2)float640.0 1.0
- long_name :
- tensor2
- units :
array([0., 1.])
- Time()timedelta64[ns]06:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array(21600000000000, dtype='timedelta64[ns]')
- dxt(xt)float64...
- long_name :
- Zonal T-grid spacing
- units :
- m
[200 values with dtype=float64]
- dxu(xu)float64...
- long_name :
- Zonal U-grid spacing
- units :
- m
[200 values with dtype=float64]
- dyt()float64...
- long_name :
- Meridional T-grid spacing
- units :
- m
[1 values with dtype=float64]
- dyu()float64...
- long_name :
- Meridional U-grid spacing
- units :
- m
[1 values with dtype=float64]
- dzt(zt)float64...
- long_name :
- Vertical spacing (T)
- units :
- m
[90 values with dtype=float64]
- dzw(zw)float64...
- long_name :
- Vertical spacing (W)
- units :
- m
[90 values with dtype=float64]
- ht(xt)float64...
- long_name :
- Total depth (T)
- units :
- m
[200 values with dtype=float64]
- hu(xu)float64...
- long_name :
- Total depth (U)
- units :
- m
[200 values with dtype=float64]
- hv(xt)float64...
- long_name :
- Total depth (V)
- units :
- m
[200 values with dtype=float64]
- beta(xt)float64...
- long_name :
- Change of Coriolis freq.
- units :
- 1/(ms)
[200 values with dtype=float64]
- area_t(xt)float64...
- long_name :
- Area of T-box
- units :
- m^2
[200 values with dtype=float64]
- area_u(xu)float64...
- long_name :
- Area of U-box
- units :
- m^2
[200 values with dtype=float64]
- area_v(xt)float64...
- long_name :
- Area of V-box
- units :
- m^2
[200 values with dtype=float64]
- rho(zt, xt)float64...
- long_name :
- Density
- units :
- kg/m^3
[18000 values with dtype=float64]
- prho(zt, xt)float64...
- long_name :
- Potential density
- units :
- kg/m^3
[18000 values with dtype=float64]
- int_drhodT(zt, xt)float64...
- long_name :
- Der. of dyn. enthalpy by temperature
- units :
- kg / (m^2 deg C)
[18000 values with dtype=float64]
- int_drhodS(zt, xt)float64...
- long_name :
- Der. of dyn. enthalpy by salinity
- units :
- kg / (m^2 g / kg)
[18000 values with dtype=float64]
- Nsqr(zw, xt)float64...
- long_name :
- Square of stability frequency
- units :
- 1/s^2
[18000 values with dtype=float64]
- Hd(zt, xt)float64...
- long_name :
- Dynamic enthalpy
- units :
- m^2/s^2
[18000 values with dtype=float64]
- temp(zt, xt)float64...
- long_name :
- Temperature
- units :
- deg C
[18000 values with dtype=float64]
- salt(zt, xt)float64...
- long_name :
- Salinity
- units :
- g/kg
[18000 values with dtype=float64]
- forc_temp_surface(xt)float64...
- long_name :
- Surface temperature flux
- units :
- m deg C/s
[200 values with dtype=float64]
- forc_salt_surface(xt)float64...
- long_name :
- Surface salinity flux
- units :
- m g/s kg
[200 values with dtype=float64]
- u(zt, xu)float64...
- long_name :
- Zonal velocity
- units :
- m/s
[18000 values with dtype=float64]
- v(zt, xt)float64...
- long_name :
- Meridional velocity
- units :
- m/s
[18000 values with dtype=float64]
- w(zw, xt)float64...
- long_name :
- Vertical velocity
- units :
- m/s
[18000 values with dtype=float64]
- p_hydro(zt, xt)float64...
- long_name :
- Hydrostatic pressure
- units :
- m^2/s^2
[18000 values with dtype=float64]
- kappaM(zt, xt)float64...
- long_name :
- Vertical viscosity
- units :
- m^2/s
[18000 values with dtype=float64]
- kappaH(zw, xt)float64...
- long_name :
- Vertical diffusivity
- units :
- m^2/s
[18000 values with dtype=float64]
- surface_taux(xu)float64...
- long_name :
- Surface wind stress
- units :
- N/m^2
[200 values with dtype=float64]
- surface_tauy(xt)float64...
- long_name :
- Surface wind stress
- units :
- N/m^2
[200 values with dtype=float64]
- forc_rho_surface(xt)float64...
- long_name :
- Surface density flux
- units :
- kg / (m^2 s)
[200 values with dtype=float64]
- psi(xt)float64...
- long_name :
- Surface pressure
- units :
- m^2/s^2
[200 values with dtype=float64]
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- xuPandasIndex
PandasIndex(Float64Index([ -149.5829291388837, -146.11747691514034, -142.656286565749, -139.19994298483698, -135.74910906034242, -132.3045354026015, -128.86707108785163, -125.43767546229275, -122.0174310337388, -118.60755745098363, ... 122.01743103373877, 125.43767546229269, 128.86707108785154, 132.3045354026014, 135.74910906034236, 139.19994298483692, 142.65628656574899, 146.11747691514034, 149.5829291388837, 153.05212756523898], dtype='float64', name='xu', length=200))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
- zwPandasIndex
PandasIndex(Float64Index([-197.77777777777814, -195.5555555555559, -193.33333333333368, -191.11111111111148, -188.88888888888926, -186.66666666666703, -184.4444444444448, -182.2222222222226, -180.00000000000037, -177.77777777777814, -175.5555555555559, -173.3333333333337, -171.11111111111148, -168.88888888888926, -166.66666666666703, -164.4444444444448, -162.2222222222226, -160.00000000000037, -157.77777777777814, -155.55555555555594, -153.3333333333337, -151.11111111111148, -148.88888888888926, -146.66666666666703, -144.44444444444483, -142.2222222222226, -140.00000000000037, -137.77777777777817, -135.55555555555594, -133.3333333333337, -131.11111111111148, -128.88888888888926, -126.66666666666703, -124.4444444444448, -122.22222222222257, -120.00000000000034, -117.77777777777811, -115.55555555555588, -113.33333333333366, -111.11111111111143, -108.8888888888892, -106.66666666666697, -104.44444444444474, -102.22222222222251, -100.00000000000028, -97.77777777777806, -95.55555555555583, -93.3333333333336, -91.11111111111137, -88.88888888888914, -86.66666666666691, -84.44444444444468, -82.22222222222246, -80.00000000000023, -77.777777777778, -75.55555555555577, -73.33333333333354, -71.11111111111131, -68.88888888888908, -66.66666666666686, -64.44444444444463, -62.2222222222224, -60.00000000000017, -57.77777777777794, -55.55555555555571, -53.333333333333485, -51.111111111111256, -48.88888888888903, -46.6666666666668, -44.44444444444457, -42.22222222222234, -40.000000000000114, -37.777777777777885, -35.55555555555566, -33.33333333333343, -31.1111111111112, -28.88888888888897, -26.666666666666742, -24.444444444444514, -22.222222222222285, -20.000000000000057, -17.77777777777783, -15.5555555555556, -13.333333333333371, -11.111111111111143, -8.888888888888914, -6.666666666666686, -4.444444444444457, -2.2222222222222285, 0.0], dtype='float64', name='zw'))
- tensor1PandasIndex
PandasIndex(Float64Index([0.0, 1.0], dtype='float64', name='tensor1'))
- tensor2PandasIndex
PandasIndex(Float64Index([0.0, 1.0], dtype='float64', name='tensor2'))
- date_created :
- 2023-09-07T15:00:26.245784
- veros_version :
- 1.5.0
- setup_identifier :
- example
- setup_description :
- setup_settings :
- {"identifier": "example", "description": "", "nx": 200, "ny": 4, "nz": 90, "dt_mom": 20.0, "dt_tracer": 20.0, "runlen": 22320.0, "AB_eps": 0.1, "x_origin": -149582.92913888372, "y_origin": 0.0, "pi": 3.141592653589793, "radius": 6370000.0, "degtom": 111177.4733520388, "omega": 7.292123516990375e-05, "rho_0": 1024.0, "grav": 9.81, "coord_degree": false, "enable_cyclic_x": false, "eq_of_state_type": 1, "enable_implicit_vert_friction": true, "enable_explicit_vert_friction": false, "enable_hor_friction": true, "enable_hor_diffusion": true, "enable_biharmonic_friction": false, "enable_biharmonic_mixing": false, "enable_hor_friction_cos_scaling": false, "enable_ray_friction": false, "enable_bottom_friction": false, "enable_bottom_friction_var": false, "enable_quadratic_bottom_friction": true, "enable_tempsalt_sources": false, "enable_momentum_sources": false, "enable_superbee_advection": false, "enable_conserve_energy": true, "enable_store_bottom_friction_tke": false, "enable_store_cabbeling_heat": false, "enable_noslip_lateral": false, "enable_streamfunction": false, "A_h": 10.0, "K_h": 10.0, "r_ray": 0.0, "r_bot": 0.0, "r_quad_bot": 0.001, "hor_friction_cosPower": 1.0, "A_hbi": 0.0, "K_hbi": 0.0, "biharmonic_friction_cosPower": 0.0, "kappaH_0": 0.0004, "kappaM_0": 0.0004, "enable_neutral_diffusion": false, "enable_skew_diffusion": false, "enable_TEM_friction": false, "K_iso_0": 0.0, "K_iso_steep": 0.0, "K_gm_0": 0.0, "iso_dslope": 0.0008, "iso_slopec": 0.001, "enable_idemix": false, "tau_v": 172800.0, "tau_h": 1296000.0, "gamma": 1.57, "jstar": 5.0, "mu0": 0.3333333333333333, "enable_idemix_hor_diffusion": false, "enable_eke_diss_bottom": false, "enable_eke_diss_surfbot": false, "eke_diss_surfbot_frac": 1.0, "enable_idemix_superbee_advection": false, "enable_idemix_upwind_advection": false, "enable_tke": false, "c_k": 0.1, "c_eps": 0.7, "alpha_tke": 1.0, "mxl_min": 1e-12, "kappaM_min": 0.0, "kappaM_max": 100.0, "tke_mxl_choice": 1, "enable_tke_superbee_advection": false, "enable_tke_upwind_advection": false, "enable_tke_hor_diffusion": false, "K_h_tke": 2000.0, "enable_eke": false, "eke_lmin": 100.0, "eke_c_k": 1.0, "eke_cross": 1.0, "eke_crhin": 1.0, "eke_c_eps": 1.0, "eke_k_max": 10000.0, "alpha_eke": 1.0, "enable_eke_superbee_advection": false, "enable_eke_upwind_advection": false, "enable_eke_isopycnal_diffusion": false, "restart_input_filename": null, "restart_output_filename": "{identifier}_{itt:0>4d}.restart.h5", "restart_frequency": 0.0, "kappaH_min": 0.0, "enable_kappaH_profile": false, "enable_Prandtl_tke": true, "Prandtl_tke0": 10.0}
- setup_file :
- /Users/jklymak/Dropbox/Teaching/Eos431Phy441/site/computing/example.py
- setup_code :
- #!/usr/bin/env python """ """ __VEROS_VERSION__ = '1.5.0' if __name__ == "__main__": raise RuntimeError( "Veros setups cannot be executed directly. " f"Try `veros run {__file__}` instead." ) # -- end of auto-generated header, original file below -- from veros import VerosSetup, veros_routine from veros.variables import allocate, Variable from veros.distributed import global_min, global_max, proc_index_to_rank, proc_rank_to_index from veros.core.operators import numpy as npx, update, at from veros.tools.setup import get_stretched_grid_steps from veros import runtime_settings as rs, runtime_state as rst class ChannelSetup(VerosSetup): """A model using cartesian coordinates in cyclic channel Wind forcing over a channel; no buoyancy """ @veros_routine def set_parameter(self, state): settings = state.settings settings.identifier = "example" settings.nx, settings.ny, settings.nz = 200, 4, 90 settings.dt_mom = 20 settings.dt_tracer = settings.dt_mom settings.runlen = 3600 * 6.2 settings.x_origin = 0.0 settings.y_origin = 0.0 settings.coord_degree = False settings.enable_cyclic_x = False settings.enable_neutral_diffusion = False settings.enable_hor_friction = True settings.enable_hor_diffusion = True settings.A_h = 10 settings.K_h = settings.A_h settings.enable_hor_friction_cos_scaling = False settings.hor_friction_cosPower = 1 settings.enable_quadratic_bottom_friction = True settings.r_quad_bot = 1e-3 settings.enable_implicit_vert_friction = True settings.enable_explicit_vert_friction = False settings.kappaM_0 = 4e-4 settings.kappaH_0 = 4e-4 settings.enable_streamfunction = False settings.enable_idemix = False settings.eq_of_state_type = 1 @veros_routine(dist_safe=False, local_variables=['dxt', 'dyt', 'dzt']) def set_grid(self, state): vs = state.variables settings = state.settings proc_idx = proc_rank_to_index(rst.proc_rank) print(proc_idx) ddz = 200 / settings.nz print(npx.shape(vs.dxt)) if False: vs.dxt = update(vs.dxt, at[...], 400) if True: for i in range(int(settings.nx / 3), -1, -1): vs.dxt = update(vs.dxt, at[i], vs.dxt[i+1] * 1.05) for i in range(int(2*settings.nx / 3)-1, settings.nx+4): vs.dxt = update(vs.dxt, at[i], vs.dxt[i-1] * 1.05) dx = get_stretched_grid_steps(102-25, 150e3, 400) vs.dxt = update(vs.dxt, at[...], npx.hstack((dx[::-1], npx.ones(50)*400, dx))) vs.dyt = update(vs.dyt, at[...], 1000) vs.dzt = update(vs.dzt, at[...], ddz) @veros_routine def set_coriolis(self, state): vs = state.variables # settings = state.settings vs.coriolis_t = update( vs.coriolis_t, at[...], 0e-4 ) @veros_routine(dist_safe=False, local_variables=['kbot', 'xt']) def set_topography(self, state): vs = state.variables # depth of deepest cell. 0 is land, 1 is deepest # nz is shallowest.. vs.kbot = update(vs.kbot, at[...], 1) kbot = npx.ones_like(vs.kbot[:, 0]) x = vs.xt x = x - npx.mean(x) kbot += npx.floor(35*npx.exp(-(x/25000)**2)).astype("int") for j in range(state.settings.ny+1): vs.kbot = update(vs.kbot, at[:, j], kbot) vs.kbot = update(vs.kbot, at[:, 0], 0) vs.kbot = update(vs.kbot, at[0, :], 0) @veros_routine(dist_safe=False, local_variables=['xu', 'xt', 'temp']) def set_initial_conditions(self, state): vs = state.variables settings = state.settings nx = settings.nx tbot = 10 ttop = 14 inx = npx.nonzero(vs.xt > npx.mean(vs.xt))[0] with settings.unlock(): settings.x_origin = -npx.mean(vs.xt) vs.xt = update(vs.xt, at[...], vs.xt+settings.x_origin) vs.xu = update(vs.xu, at[...], vs.xu+settings.x_origin) vs.temp = update(vs.temp, at[:inx[0], :, :, :], tbot) vs.temp = update(vs.temp, at[inx[0]:, :, :, :], ttop) vs.temp = update(vs.temp, at[:, :, :30, :], tbot) vs.temp = update(vs.temp, at[:, :, settings.nz-30:, :], ttop) @veros_routine def set_forcing(self, state): pass # vs = state.variables # vs.forc_temp_surface = vs.t_rest * (vs.t_star - vs.temp[:, :, -1, vs.tau]) @veros_routine def set_diagnostics(self, state): settings = state.settings diagnostics = state.diagnostics diagnostics["snapshot"].output_frequency = 7200 / 2 @veros_routine def after_timestep(self, state): pass
But if we do not choose a value that is exactly in the coordinate we can get an error:
# ignore this "xmode" magic; it just makes the Error message _much_ shorter below
%xmode Minimal
with xr.open_dataset('example.snapshot.nc') as ds:
# time is 370 minutes, or just a bit off from 6 h where we have data:
ds = ds.isel(yt=0, yu=0).sel(Time=np.timedelta64(6*60+10, 'm'))
Exception reporting mode: Minimal
KeyError: 22200000000000
During handling of the above exception, another exception occurred:
KeyError: Timedelta('0 days 06:10:00')
The above exception was the direct cause of the following exception:
KeyError: Timedelta('0 days 06:10:00')
The above exception was the direct cause of the following exception:
KeyError: "not all values found in index 'Time'. Try setting the `method` keyword argument (example: method='nearest')."
In this case we can still proceed using the error message above as guidance:
with xr.open_dataset('example.snapshot.nc') as ds:
# time is 370 minutes, or just a bit off from 6 h where we have data:
ds = ds.isel(yt=0, yu=0).sel(Time=np.timedelta64(6*60+10, 'm'), method='nearest')
display(ds)
<xarray.Dataset> Dimensions: (xt: 200, xu: 200, zt: 90, zw: 90, tensor1: 2, tensor2: 2) Coordinates: (9) Data variables: (12/33) dxt (xt) float64 ... dxu (xu) float64 ... dyt float64 ... dyu float64 ... dzt (zt) float64 ... dzw (zw) float64 ... ... ... kappaM (zt, xt) float64 ... kappaH (zw, xt) float64 ... surface_taux (xu) float64 ... surface_tauy (xt) float64 ... forc_rho_surface (xt) float64 ... psi (xt) float64 ... Attributes: (7)
- xt: 200
- xu: 200
- zt: 90
- zw: 90
- tensor1: 2
- tensor2: 2
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- xu(xu)float64-149.6 -146.1 ... 149.6 153.1
- long_name :
- Zonal coordinate (U)
- units :
- km
array([-1.495829e+02, -1.461175e+02, -1.426563e+02, -1.391999e+02, -1.357491e+02, -1.323045e+02, -1.288671e+02, -1.254377e+02, -1.220174e+02, -1.186076e+02, -1.152094e+02, -1.118246e+02, -1.084547e+02, -1.051018e+02, -1.017680e+02, -9.845565e+01, -9.516740e+01, -9.190616e+01, -8.867512e+01, -8.547776e+01, -8.231785e+01, -7.919946e+01, -7.612691e+01, -7.310480e+01, -7.013796e+01, -6.723138e+01, -6.439016e+01, -6.161946e+01, -5.892438e+01, -5.630987e+01, -5.378062e+01, -5.134095e+01, -4.899467e+01, -4.674498e+01, -4.459441e+01, -4.254466e+01, -4.059661e+01, -3.875025e+01, -3.700472e+01, -3.535830e+01, -3.380848e+01, -3.235205e+01, -3.098520e+01, -2.970360e+01, -2.850258e+01, -2.737717e+01, -2.632228e+01, -2.533277e+01, -2.440350e+01, -2.352950e+01, -2.270595e+01, -2.192824e+01, -2.119205e+01, -2.049330e+01, -1.982824e+01, -1.919337e+01, -1.858552e+01, -1.800177e+01, -1.743949e+01, -1.689630e+01, -1.637003e+01, -1.585878e+01, -1.536080e+01, -1.487457e+01, -1.439871e+01, -1.393201e+01, -1.347337e+01, -1.302184e+01, -1.257657e+01, -1.213681e+01, -1.170189e+01, -1.127124e+01, -1.084434e+01, -1.042072e+01, -1.000000e+01, -9.600000e+00, -9.200000e+00, -8.800000e+00, -8.400000e+00, -8.000000e+00, -7.600000e+00, -7.200000e+00, -6.800000e+00, -6.400000e+00, -6.000000e+00, -5.600000e+00, -5.200000e+00, -4.800000e+00, -4.400000e+00, -4.000000e+00, -3.600000e+00, -3.200000e+00, -2.800000e+00, -2.400000e+00, -2.000000e+00, -1.600000e+00, -1.200000e+00, -8.000000e-01, -4.000000e-01, 2.910383e-14, 4.000000e-01, 8.000000e-01, 1.200000e+00, 1.600000e+00, 2.000000e+00, 2.400000e+00, 2.800000e+00, 3.200000e+00, 3.600000e+00, 4.000000e+00, 4.400000e+00, 4.800000e+00, 5.200000e+00, 5.600000e+00, 6.000000e+00, 6.400000e+00, 6.800000e+00, 7.200000e+00, 7.600000e+00, 8.000000e+00, 8.400000e+00, 8.800000e+00, 9.200000e+00, 9.600000e+00, 1.000000e+01, 1.042072e+01, 1.084434e+01, 1.127124e+01, 1.170189e+01, 1.213681e+01, 1.257657e+01, 1.302184e+01, 1.347337e+01, 1.393201e+01, 1.439871e+01, 1.487457e+01, 1.536080e+01, 1.585878e+01, 1.637003e+01, 1.689630e+01, 1.743949e+01, 1.800177e+01, 1.858552e+01, 1.919337e+01, 1.982824e+01, 2.049330e+01, 2.119205e+01, 2.192824e+01, 2.270595e+01, 2.352950e+01, 2.440350e+01, 2.533277e+01, 2.632228e+01, 2.737717e+01, 2.850258e+01, 2.970360e+01, 3.098520e+01, 3.235205e+01, 3.380848e+01, 3.535830e+01, 3.700472e+01, 3.875025e+01, 4.059661e+01, 4.254466e+01, 4.459441e+01, 4.674498e+01, 4.899467e+01, 5.134095e+01, 5.378062e+01, 5.630987e+01, 5.892438e+01, 6.161946e+01, 6.439016e+01, 6.723138e+01, 7.013796e+01, 7.310480e+01, 7.612691e+01, 7.919946e+01, 8.231785e+01, 8.547776e+01, 8.867512e+01, 9.190616e+01, 9.516740e+01, 9.845565e+01, 1.017680e+02, 1.051018e+02, 1.084547e+02, 1.118246e+02, 1.152094e+02, 1.186076e+02, 1.220174e+02, 1.254377e+02, 1.288671e+02, 1.323045e+02, 1.357491e+02, 1.391999e+02, 1.426563e+02, 1.461175e+02, 1.495829e+02, 1.530521e+02])
- yt()float64-0.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array(-0.5)
- yu()float640.0
- long_name :
- Meridional coordinate (U)
- units :
- km
array(0.)
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- zw(zw)float64-197.8 -195.6 -193.3 ... -2.222 0.0
- long_name :
- Vertical coordinate (W)
- units :
- m
- positive :
- up
array([-197.777778, -195.555556, -193.333333, -191.111111, -188.888889, -186.666667, -184.444444, -182.222222, -180. , -177.777778, -175.555556, -173.333333, -171.111111, -168.888889, -166.666667, -164.444444, -162.222222, -160. , -157.777778, -155.555556, -153.333333, -151.111111, -148.888889, -146.666667, -144.444444, -142.222222, -140. , -137.777778, -135.555556, -133.333333, -131.111111, -128.888889, -126.666667, -124.444444, -122.222222, -120. , -117.777778, -115.555556, -113.333333, -111.111111, -108.888889, -106.666667, -104.444444, -102.222222, -100. , -97.777778, -95.555556, -93.333333, -91.111111, -88.888889, -86.666667, -84.444444, -82.222222, -80. , -77.777778, -75.555556, -73.333333, -71.111111, -68.888889, -66.666667, -64.444444, -62.222222, -60. , -57.777778, -55.555556, -53.333333, -51.111111, -48.888889, -46.666667, -44.444444, -42.222222, -40. , -37.777778, -35.555556, -33.333333, -31.111111, -28.888889, -26.666667, -24.444444, -22.222222, -20. , -17.777778, -15.555556, -13.333333, -11.111111, -8.888889, -6.666667, -4.444444, -2.222222, 0. ])
- tensor1(tensor1)float640.0 1.0
- long_name :
- tensor1
- units :
array([0., 1.])
- tensor2(tensor2)float640.0 1.0
- long_name :
- tensor2
- units :
array([0., 1.])
- Time()timedelta64[ns]06:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array(21600000000000, dtype='timedelta64[ns]')
- dxt(xt)float64...
- long_name :
- Zonal T-grid spacing
- units :
- m
[200 values with dtype=float64]
- dxu(xu)float64...
- long_name :
- Zonal U-grid spacing
- units :
- m
[200 values with dtype=float64]
- dyt()float64...
- long_name :
- Meridional T-grid spacing
- units :
- m
[1 values with dtype=float64]
- dyu()float64...
- long_name :
- Meridional U-grid spacing
- units :
- m
[1 values with dtype=float64]
- dzt(zt)float64...
- long_name :
- Vertical spacing (T)
- units :
- m
[90 values with dtype=float64]
- dzw(zw)float64...
- long_name :
- Vertical spacing (W)
- units :
- m
[90 values with dtype=float64]
- ht(xt)float64...
- long_name :
- Total depth (T)
- units :
- m
[200 values with dtype=float64]
- hu(xu)float64...
- long_name :
- Total depth (U)
- units :
- m
[200 values with dtype=float64]
- hv(xt)float64...
- long_name :
- Total depth (V)
- units :
- m
[200 values with dtype=float64]
- beta(xt)float64...
- long_name :
- Change of Coriolis freq.
- units :
- 1/(ms)
[200 values with dtype=float64]
- area_t(xt)float64...
- long_name :
- Area of T-box
- units :
- m^2
[200 values with dtype=float64]
- area_u(xu)float64...
- long_name :
- Area of U-box
- units :
- m^2
[200 values with dtype=float64]
- area_v(xt)float64...
- long_name :
- Area of V-box
- units :
- m^2
[200 values with dtype=float64]
- rho(zt, xt)float64...
- long_name :
- Density
- units :
- kg/m^3
[18000 values with dtype=float64]
- prho(zt, xt)float64...
- long_name :
- Potential density
- units :
- kg/m^3
[18000 values with dtype=float64]
- int_drhodT(zt, xt)float64...
- long_name :
- Der. of dyn. enthalpy by temperature
- units :
- kg / (m^2 deg C)
[18000 values with dtype=float64]
- int_drhodS(zt, xt)float64...
- long_name :
- Der. of dyn. enthalpy by salinity
- units :
- kg / (m^2 g / kg)
[18000 values with dtype=float64]
- Nsqr(zw, xt)float64...
- long_name :
- Square of stability frequency
- units :
- 1/s^2
[18000 values with dtype=float64]
- Hd(zt, xt)float64...
- long_name :
- Dynamic enthalpy
- units :
- m^2/s^2
[18000 values with dtype=float64]
- temp(zt, xt)float64...
- long_name :
- Temperature
- units :
- deg C
[18000 values with dtype=float64]
- salt(zt, xt)float64...
- long_name :
- Salinity
- units :
- g/kg
[18000 values with dtype=float64]
- forc_temp_surface(xt)float64...
- long_name :
- Surface temperature flux
- units :
- m deg C/s
[200 values with dtype=float64]
- forc_salt_surface(xt)float64...
- long_name :
- Surface salinity flux
- units :
- m g/s kg
[200 values with dtype=float64]
- u(zt, xu)float64...
- long_name :
- Zonal velocity
- units :
- m/s
[18000 values with dtype=float64]
- v(zt, xt)float64...
- long_name :
- Meridional velocity
- units :
- m/s
[18000 values with dtype=float64]
- w(zw, xt)float64...
- long_name :
- Vertical velocity
- units :
- m/s
[18000 values with dtype=float64]
- p_hydro(zt, xt)float64...
- long_name :
- Hydrostatic pressure
- units :
- m^2/s^2
[18000 values with dtype=float64]
- kappaM(zt, xt)float64...
- long_name :
- Vertical viscosity
- units :
- m^2/s
[18000 values with dtype=float64]
- kappaH(zw, xt)float64...
- long_name :
- Vertical diffusivity
- units :
- m^2/s
[18000 values with dtype=float64]
- surface_taux(xu)float64...
- long_name :
- Surface wind stress
- units :
- N/m^2
[200 values with dtype=float64]
- surface_tauy(xt)float64...
- long_name :
- Surface wind stress
- units :
- N/m^2
[200 values with dtype=float64]
- forc_rho_surface(xt)float64...
- long_name :
- Surface density flux
- units :
- kg / (m^2 s)
[200 values with dtype=float64]
- psi(xt)float64...
- long_name :
- Surface pressure
- units :
- m^2/s^2
[200 values with dtype=float64]
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- xuPandasIndex
PandasIndex(Float64Index([ -149.5829291388837, -146.11747691514034, -142.656286565749, -139.19994298483698, -135.74910906034242, -132.3045354026015, -128.86707108785163, -125.43767546229275, -122.0174310337388, -118.60755745098363, ... 122.01743103373877, 125.43767546229269, 128.86707108785154, 132.3045354026014, 135.74910906034236, 139.19994298483692, 142.65628656574899, 146.11747691514034, 149.5829291388837, 153.05212756523898], dtype='float64', name='xu', length=200))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
- zwPandasIndex
PandasIndex(Float64Index([-197.77777777777814, -195.5555555555559, -193.33333333333368, -191.11111111111148, -188.88888888888926, -186.66666666666703, -184.4444444444448, -182.2222222222226, -180.00000000000037, -177.77777777777814, -175.5555555555559, -173.3333333333337, -171.11111111111148, -168.88888888888926, -166.66666666666703, -164.4444444444448, -162.2222222222226, -160.00000000000037, -157.77777777777814, -155.55555555555594, -153.3333333333337, -151.11111111111148, -148.88888888888926, -146.66666666666703, -144.44444444444483, -142.2222222222226, -140.00000000000037, -137.77777777777817, -135.55555555555594, -133.3333333333337, -131.11111111111148, -128.88888888888926, -126.66666666666703, -124.4444444444448, -122.22222222222257, -120.00000000000034, -117.77777777777811, -115.55555555555588, -113.33333333333366, -111.11111111111143, -108.8888888888892, -106.66666666666697, -104.44444444444474, -102.22222222222251, -100.00000000000028, -97.77777777777806, -95.55555555555583, -93.3333333333336, -91.11111111111137, -88.88888888888914, -86.66666666666691, -84.44444444444468, -82.22222222222246, -80.00000000000023, -77.777777777778, -75.55555555555577, -73.33333333333354, -71.11111111111131, -68.88888888888908, -66.66666666666686, -64.44444444444463, -62.2222222222224, -60.00000000000017, -57.77777777777794, -55.55555555555571, -53.333333333333485, -51.111111111111256, -48.88888888888903, -46.6666666666668, -44.44444444444457, -42.22222222222234, -40.000000000000114, -37.777777777777885, -35.55555555555566, -33.33333333333343, -31.1111111111112, -28.88888888888897, -26.666666666666742, -24.444444444444514, -22.222222222222285, -20.000000000000057, -17.77777777777783, -15.5555555555556, -13.333333333333371, -11.111111111111143, -8.888888888888914, -6.666666666666686, -4.444444444444457, -2.2222222222222285, 0.0], dtype='float64', name='zw'))
- tensor1PandasIndex
PandasIndex(Float64Index([0.0, 1.0], dtype='float64', name='tensor1'))
- tensor2PandasIndex
PandasIndex(Float64Index([0.0, 1.0], dtype='float64', name='tensor2'))
- date_created :
- 2023-09-07T15:00:26.245784
- veros_version :
- 1.5.0
- setup_identifier :
- example
- setup_description :
- setup_settings :
- {"identifier": "example", "description": "", "nx": 200, "ny": 4, "nz": 90, "dt_mom": 20.0, "dt_tracer": 20.0, "runlen": 22320.0, "AB_eps": 0.1, "x_origin": -149582.92913888372, "y_origin": 0.0, "pi": 3.141592653589793, "radius": 6370000.0, "degtom": 111177.4733520388, "omega": 7.292123516990375e-05, "rho_0": 1024.0, "grav": 9.81, "coord_degree": false, "enable_cyclic_x": false, "eq_of_state_type": 1, "enable_implicit_vert_friction": true, "enable_explicit_vert_friction": false, "enable_hor_friction": true, "enable_hor_diffusion": true, "enable_biharmonic_friction": false, "enable_biharmonic_mixing": false, "enable_hor_friction_cos_scaling": false, "enable_ray_friction": false, "enable_bottom_friction": false, "enable_bottom_friction_var": false, "enable_quadratic_bottom_friction": true, "enable_tempsalt_sources": false, "enable_momentum_sources": false, "enable_superbee_advection": false, "enable_conserve_energy": true, "enable_store_bottom_friction_tke": false, "enable_store_cabbeling_heat": false, "enable_noslip_lateral": false, "enable_streamfunction": false, "A_h": 10.0, "K_h": 10.0, "r_ray": 0.0, "r_bot": 0.0, "r_quad_bot": 0.001, "hor_friction_cosPower": 1.0, "A_hbi": 0.0, "K_hbi": 0.0, "biharmonic_friction_cosPower": 0.0, "kappaH_0": 0.0004, "kappaM_0": 0.0004, "enable_neutral_diffusion": false, "enable_skew_diffusion": false, "enable_TEM_friction": false, "K_iso_0": 0.0, "K_iso_steep": 0.0, "K_gm_0": 0.0, "iso_dslope": 0.0008, "iso_slopec": 0.001, "enable_idemix": false, "tau_v": 172800.0, "tau_h": 1296000.0, "gamma": 1.57, "jstar": 5.0, "mu0": 0.3333333333333333, "enable_idemix_hor_diffusion": false, "enable_eke_diss_bottom": false, "enable_eke_diss_surfbot": false, "eke_diss_surfbot_frac": 1.0, "enable_idemix_superbee_advection": false, "enable_idemix_upwind_advection": false, "enable_tke": false, "c_k": 0.1, "c_eps": 0.7, "alpha_tke": 1.0, "mxl_min": 1e-12, "kappaM_min": 0.0, "kappaM_max": 100.0, "tke_mxl_choice": 1, "enable_tke_superbee_advection": false, "enable_tke_upwind_advection": false, "enable_tke_hor_diffusion": false, "K_h_tke": 2000.0, "enable_eke": false, "eke_lmin": 100.0, "eke_c_k": 1.0, "eke_cross": 1.0, "eke_crhin": 1.0, "eke_c_eps": 1.0, "eke_k_max": 10000.0, "alpha_eke": 1.0, "enable_eke_superbee_advection": false, "enable_eke_upwind_advection": false, "enable_eke_isopycnal_diffusion": false, "restart_input_filename": null, "restart_output_filename": "{identifier}_{itt:0>4d}.restart.h5", "restart_frequency": 0.0, "kappaH_min": 0.0, "enable_kappaH_profile": false, "enable_Prandtl_tke": true, "Prandtl_tke0": 10.0}
- setup_file :
- /Users/jklymak/Dropbox/Teaching/Eos431Phy441/site/computing/example.py
- setup_code :
- #!/usr/bin/env python """ """ __VEROS_VERSION__ = '1.5.0' if __name__ == "__main__": raise RuntimeError( "Veros setups cannot be executed directly. " f"Try `veros run {__file__}` instead." ) # -- end of auto-generated header, original file below -- from veros import VerosSetup, veros_routine from veros.variables import allocate, Variable from veros.distributed import global_min, global_max, proc_index_to_rank, proc_rank_to_index from veros.core.operators import numpy as npx, update, at from veros.tools.setup import get_stretched_grid_steps from veros import runtime_settings as rs, runtime_state as rst class ChannelSetup(VerosSetup): """A model using cartesian coordinates in cyclic channel Wind forcing over a channel; no buoyancy """ @veros_routine def set_parameter(self, state): settings = state.settings settings.identifier = "example" settings.nx, settings.ny, settings.nz = 200, 4, 90 settings.dt_mom = 20 settings.dt_tracer = settings.dt_mom settings.runlen = 3600 * 6.2 settings.x_origin = 0.0 settings.y_origin = 0.0 settings.coord_degree = False settings.enable_cyclic_x = False settings.enable_neutral_diffusion = False settings.enable_hor_friction = True settings.enable_hor_diffusion = True settings.A_h = 10 settings.K_h = settings.A_h settings.enable_hor_friction_cos_scaling = False settings.hor_friction_cosPower = 1 settings.enable_quadratic_bottom_friction = True settings.r_quad_bot = 1e-3 settings.enable_implicit_vert_friction = True settings.enable_explicit_vert_friction = False settings.kappaM_0 = 4e-4 settings.kappaH_0 = 4e-4 settings.enable_streamfunction = False settings.enable_idemix = False settings.eq_of_state_type = 1 @veros_routine(dist_safe=False, local_variables=['dxt', 'dyt', 'dzt']) def set_grid(self, state): vs = state.variables settings = state.settings proc_idx = proc_rank_to_index(rst.proc_rank) print(proc_idx) ddz = 200 / settings.nz print(npx.shape(vs.dxt)) if False: vs.dxt = update(vs.dxt, at[...], 400) if True: for i in range(int(settings.nx / 3), -1, -1): vs.dxt = update(vs.dxt, at[i], vs.dxt[i+1] * 1.05) for i in range(int(2*settings.nx / 3)-1, settings.nx+4): vs.dxt = update(vs.dxt, at[i], vs.dxt[i-1] * 1.05) dx = get_stretched_grid_steps(102-25, 150e3, 400) vs.dxt = update(vs.dxt, at[...], npx.hstack((dx[::-1], npx.ones(50)*400, dx))) vs.dyt = update(vs.dyt, at[...], 1000) vs.dzt = update(vs.dzt, at[...], ddz) @veros_routine def set_coriolis(self, state): vs = state.variables # settings = state.settings vs.coriolis_t = update( vs.coriolis_t, at[...], 0e-4 ) @veros_routine(dist_safe=False, local_variables=['kbot', 'xt']) def set_topography(self, state): vs = state.variables # depth of deepest cell. 0 is land, 1 is deepest # nz is shallowest.. vs.kbot = update(vs.kbot, at[...], 1) kbot = npx.ones_like(vs.kbot[:, 0]) x = vs.xt x = x - npx.mean(x) kbot += npx.floor(35*npx.exp(-(x/25000)**2)).astype("int") for j in range(state.settings.ny+1): vs.kbot = update(vs.kbot, at[:, j], kbot) vs.kbot = update(vs.kbot, at[:, 0], 0) vs.kbot = update(vs.kbot, at[0, :], 0) @veros_routine(dist_safe=False, local_variables=['xu', 'xt', 'temp']) def set_initial_conditions(self, state): vs = state.variables settings = state.settings nx = settings.nx tbot = 10 ttop = 14 inx = npx.nonzero(vs.xt > npx.mean(vs.xt))[0] with settings.unlock(): settings.x_origin = -npx.mean(vs.xt) vs.xt = update(vs.xt, at[...], vs.xt+settings.x_origin) vs.xu = update(vs.xu, at[...], vs.xu+settings.x_origin) vs.temp = update(vs.temp, at[:inx[0], :, :, :], tbot) vs.temp = update(vs.temp, at[inx[0]:, :, :, :], ttop) vs.temp = update(vs.temp, at[:, :, :30, :], tbot) vs.temp = update(vs.temp, at[:, :, settings.nz-30:, :], ttop) @veros_routine def set_forcing(self, state): pass # vs = state.variables # vs.forc_temp_surface = vs.t_rest * (vs.t_star - vs.temp[:, :, -1, vs.tau]) @veros_routine def set_diagnostics(self, state): settings = state.settings diagnostics = state.diagnostics diagnostics["snapshot"].output_frequency = 7200 / 2 @veros_routine def after_timestep(self, state): pass
Notice that the Time that gets chosen in 06:00:00
. Of course you can do the same selection mechanisms with other coordinates.
Dealing with staggered grids#
The staggering of the variable locations (mentioned above), sometime doesn’t matter, but it is good to keep track of. It is also the source of a potential pitfall when using xarray. If the dimensions of two variables do not match, unexpected things can happen. For instance, its not terribly useful, but imagine we wanted to get the speed from the u
and v
values. We might naively do this, forgetting that the dimensions are different:
with xr.open_dataset('example.snapshot.nc') as ds:
ds = ds.isel(Time=2)
abs_speed = (ds.u**2 + ds.v**2)**(0.5)
display(abs_speed)
<xarray.DataArray (zt: 90, yt: 4, xu: 200, yu: 4, xt: 200)> 0.000186 0.000186 0.000186 0.000186 0.000186 0.000186 ... nan nan nan nan nan Coordinates: (6)
- zt: 90
- yt: 4
- xu: 200
- yu: 4
- xt: 200
- 0.000186 0.000186 0.000186 0.000186 0.000186 ... nan nan nan nan nan
array([[[[[0.00018602, 0.00018602, 0.00018602, ..., 0.00018602, 0.00018602, 0.00018602], [0.00018602, 0.00018602, 0.00018602, ..., 0.00018602, 0.00018602, 0.00018602], [0.00018602, 0.00018602, 0.00018602, ..., 0.00018602, 0.00018602, 0.00018602], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00036691, 0.00036691, 0.00036691, ..., 0.00036691, 0.00036691, 0.00036691], [0.00036691, 0.00036691, 0.00036691, ..., 0.00036691, 0.00036691, 0.00036691], [0.00036691, 0.00036691, 0.00036691, ..., 0.00036691, 0.00036691, 0.00036691], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00053812, 0.00053812, 0.00053812, ..., 0.00053812, 0.00053812, 0.00053812], ... [ nan, nan, nan, ..., nan, nan, nan]], [[0.0001844 , 0.0001844 , 0.0001844 , ..., 0.0001844 , 0.0001844 , 0.0001844 ], [0.0001844 , 0.0001844 , 0.0001844 , ..., 0.0001844 , 0.0001844 , 0.0001844 ], [0.0001844 , 0.0001844 , 0.0001844 , ..., 0.0001844 , 0.0001844 , 0.0001844 ], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]]]]])
- xu(xu)float64-149.6 -146.1 ... 149.6 153.1
- long_name :
- Zonal coordinate (U)
- units :
- km
array([-1.495829e+02, -1.461175e+02, -1.426563e+02, -1.391999e+02, -1.357491e+02, -1.323045e+02, -1.288671e+02, -1.254377e+02, -1.220174e+02, -1.186076e+02, -1.152094e+02, -1.118246e+02, -1.084547e+02, -1.051018e+02, -1.017680e+02, -9.845565e+01, -9.516740e+01, -9.190616e+01, -8.867512e+01, -8.547776e+01, -8.231785e+01, -7.919946e+01, -7.612691e+01, -7.310480e+01, -7.013796e+01, -6.723138e+01, -6.439016e+01, -6.161946e+01, -5.892438e+01, -5.630987e+01, -5.378062e+01, -5.134095e+01, -4.899467e+01, -4.674498e+01, -4.459441e+01, -4.254466e+01, -4.059661e+01, -3.875025e+01, -3.700472e+01, -3.535830e+01, -3.380848e+01, -3.235205e+01, -3.098520e+01, -2.970360e+01, -2.850258e+01, -2.737717e+01, -2.632228e+01, -2.533277e+01, -2.440350e+01, -2.352950e+01, -2.270595e+01, -2.192824e+01, -2.119205e+01, -2.049330e+01, -1.982824e+01, -1.919337e+01, -1.858552e+01, -1.800177e+01, -1.743949e+01, -1.689630e+01, -1.637003e+01, -1.585878e+01, -1.536080e+01, -1.487457e+01, -1.439871e+01, -1.393201e+01, -1.347337e+01, -1.302184e+01, -1.257657e+01, -1.213681e+01, -1.170189e+01, -1.127124e+01, -1.084434e+01, -1.042072e+01, -1.000000e+01, -9.600000e+00, -9.200000e+00, -8.800000e+00, -8.400000e+00, -8.000000e+00, -7.600000e+00, -7.200000e+00, -6.800000e+00, -6.400000e+00, -6.000000e+00, -5.600000e+00, -5.200000e+00, -4.800000e+00, -4.400000e+00, -4.000000e+00, -3.600000e+00, -3.200000e+00, -2.800000e+00, -2.400000e+00, -2.000000e+00, -1.600000e+00, -1.200000e+00, -8.000000e-01, -4.000000e-01, 2.910383e-14, 4.000000e-01, 8.000000e-01, 1.200000e+00, 1.600000e+00, 2.000000e+00, 2.400000e+00, 2.800000e+00, 3.200000e+00, 3.600000e+00, 4.000000e+00, 4.400000e+00, 4.800000e+00, 5.200000e+00, 5.600000e+00, 6.000000e+00, 6.400000e+00, 6.800000e+00, 7.200000e+00, 7.600000e+00, 8.000000e+00, 8.400000e+00, 8.800000e+00, 9.200000e+00, 9.600000e+00, 1.000000e+01, 1.042072e+01, 1.084434e+01, 1.127124e+01, 1.170189e+01, 1.213681e+01, 1.257657e+01, 1.302184e+01, 1.347337e+01, 1.393201e+01, 1.439871e+01, 1.487457e+01, 1.536080e+01, 1.585878e+01, 1.637003e+01, 1.689630e+01, 1.743949e+01, 1.800177e+01, 1.858552e+01, 1.919337e+01, 1.982824e+01, 2.049330e+01, 2.119205e+01, 2.192824e+01, 2.270595e+01, 2.352950e+01, 2.440350e+01, 2.533277e+01, 2.632228e+01, 2.737717e+01, 2.850258e+01, 2.970360e+01, 3.098520e+01, 3.235205e+01, 3.380848e+01, 3.535830e+01, 3.700472e+01, 3.875025e+01, 4.059661e+01, 4.254466e+01, 4.459441e+01, 4.674498e+01, 4.899467e+01, 5.134095e+01, 5.378062e+01, 5.630987e+01, 5.892438e+01, 6.161946e+01, 6.439016e+01, 6.723138e+01, 7.013796e+01, 7.310480e+01, 7.612691e+01, 7.919946e+01, 8.231785e+01, 8.547776e+01, 8.867512e+01, 9.190616e+01, 9.516740e+01, 9.845565e+01, 1.017680e+02, 1.051018e+02, 1.084547e+02, 1.118246e+02, 1.152094e+02, 1.186076e+02, 1.220174e+02, 1.254377e+02, 1.288671e+02, 1.323045e+02, 1.357491e+02, 1.391999e+02, 1.426563e+02, 1.461175e+02, 1.495829e+02, 1.530521e+02])
- yt(yt)float64-0.5 0.5 1.5 2.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array([-0.5, 0.5, 1.5, 2.5])
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- Time()timedelta64[ns]03:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array(10800000000000, dtype='timedelta64[ns]')
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- yu(yu)float640.0 1.0 2.0 3.0
- long_name :
- Meridional coordinate (U)
- units :
- km
array([0., 1., 2., 3.])
- xuPandasIndex
PandasIndex(Float64Index([ -149.5829291388837, -146.11747691514034, -142.656286565749, -139.19994298483698, -135.74910906034242, -132.3045354026015, -128.86707108785163, -125.43767546229275, -122.0174310337388, -118.60755745098363, ... 122.01743103373877, 125.43767546229269, 128.86707108785154, 132.3045354026014, 135.74910906034236, 139.19994298483692, 142.65628656574899, 146.11747691514034, 149.5829291388837, 153.05212756523898], dtype='float64', name='xu', length=200))
- ytPandasIndex
PandasIndex(Float64Index([-0.5, 0.5, 1.5, 2.5], dtype='float64', name='yt'))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- yuPandasIndex
PandasIndex(Float64Index([0.0, 1.0, 2.0, 3.0], dtype='float64', name='yu'))
Note that this new DataArray has three dimensions, whereas we almost certaily wanted it to just have two. Note that the new data array is also much larger than expected!
Averaging to common coordinates#
The right way to add together the data on different coordinates is to average both onto the center of the cells, which is possible directly in xarray:
ds['ucenter'] = ds.u.rolling(xu=2, center=True).mean().swap_dims({'xu':'xt'})
display(ds.ucenter)
<xarray.DataArray 'ucenter' (zt: 90, yt: 4, xt: 200)> nan -0.0002765 -0.0004525 -0.000617 ... -0.0006121 -0.0004488 -0.0002741 nan Coordinates: (4) Attributes: (2)
- zt: 90
- yt: 4
- xt: 200
- nan -0.0002765 -0.0004525 -0.000617 ... -0.0004488 -0.0002741 nan
array([[[ nan, -0.00027646, -0.00045251, ..., -0.00045124, -0.00027571, nan], [ nan, -0.00027646, -0.00045251, ..., -0.00045124, -0.00027571, nan], [ nan, -0.00027646, -0.00045251, ..., -0.00045124, -0.00027571, nan], [ nan, -0.00027646, -0.00045251, ..., -0.00045124, -0.00027571, nan]], [[ nan, -0.00027646, -0.00045251, ..., -0.00045124, -0.00027571, nan], [ nan, -0.00027646, -0.00045251, ..., -0.00045124, -0.00027571, nan], [ nan, -0.00027646, -0.00045251, ..., -0.00045124, -0.00027571, nan], [ nan, -0.00027646, -0.00045251, ..., -0.00045124, -0.00027571, nan]], [[ nan, -0.00027645, -0.00045249, ..., -0.00045121, -0.0002757 , nan], ... [ nan, -0.0002766 , -0.00045267, ..., -0.00045088, -0.00027549, nan]], [[ nan, -0.00027701, -0.0004533 , ..., -0.00045033, -0.00027512, nan], [ nan, -0.00027701, -0.0004533 , ..., -0.00045033, -0.00027512, nan], [ nan, -0.00027701, -0.0004533 , ..., -0.00045033, -0.00027512, nan], [ nan, -0.00027701, -0.0004533 , ..., -0.00045033, -0.00027512, nan]], [[ nan, -0.00027858, -0.00045577, ..., -0.00044877, -0.0002741 , nan], [ nan, -0.00027858, -0.00045577, ..., -0.00044877, -0.0002741 , nan], [ nan, -0.00027858, -0.00045577, ..., -0.00044877, -0.0002741 , nan], [ nan, -0.00027858, -0.00045577, ..., -0.00044877, -0.0002741 , nan]]])
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- yt(yt)float64-0.5 0.5 1.5 2.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array([-0.5, 0.5, 1.5, 2.5])
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- Time()timedelta64[ns]03:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array(10800000000000, dtype='timedelta64[ns]')
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- ytPandasIndex
PandasIndex(Float64Index([-0.5, 0.5, 1.5, 2.5], dtype='float64', name='yt'))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
- long_name :
- Zonal velocity
- units :
- m/s
Note there were three steps to to this process 1) create a rolling
object in the xu
dimension, and then 2) take the mean. 3) The dimensions of the new object are still xu
. We can assign the dimensions as xt
instead using swap_dims
.
Now when we calculate the speed, the dimesnions match:
ds['vcenter'] = ds.v.rolling(yu=2).mean().swap_dims({'yu':'yt'})
abs_speed = (ds.ucenter**2 + ds.vcenter**2)**(0.5)
display(abs_speed)
<xarray.DataArray (zt: 90, yt: 4, xt: 200)> nan nan nan nan nan nan nan nan nan nan ... nan nan nan nan nan nan nan nan nan Coordinates: (4)
- zt: 90
- yt: 4
- xt: 200
- nan nan nan nan nan nan nan nan ... nan nan nan nan nan nan nan nan
array([[[ nan, nan, nan, ..., nan, nan, nan], [ nan, 0.00027646, 0.00045251, ..., 0.00045124, 0.00027571, nan], [ nan, 0.00027646, 0.00045251, ..., 0.00045124, 0.00027571, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, 0.00027646, 0.00045251, ..., 0.00045124, 0.00027571, nan], [ nan, 0.00027646, 0.00045251, ..., 0.00045124, 0.00027571, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], ... [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, 0.00027701, 0.0004533 , ..., 0.00045033, 0.00027512, nan], [ nan, 0.00027701, 0.0004533 , ..., 0.00045033, 0.00027512, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[ nan, nan, nan, ..., nan, nan, nan], [ nan, 0.00027858, 0.00045577, ..., 0.00044877, 0.0002741 , nan], [ nan, 0.00027858, 0.00045577, ..., 0.00044877, 0.0002741 , nan], [ nan, nan, nan, ..., nan, nan, nan]]])
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- yt(yt)float64-0.5 0.5 1.5 2.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array([-0.5, 0.5, 1.5, 2.5])
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- Time()timedelta64[ns]03:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array(10800000000000, dtype='timedelta64[ns]')
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- ytPandasIndex
PandasIndex(Float64Index([-0.5, 0.5, 1.5, 2.5], dtype='float64', name='yt'))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
Ignoring coordinates:#
If we are not concerned with accuracy, we can also cheat and just use the values of the arrays and create a new variable:
with xr.open_dataset('example.snapshot.nc') as ds:
ds = ds.isel(Time=2)
abs_speed = (ds.u.values**2 + ds.v.values**2)**(0.5)
display(abs_speed)
array([[[0.00018602, 0.00036691, 0.00053812, ..., 0.0003659 ,
0.00018552, nan],
[0.00018602, 0.00036691, 0.00053812, ..., 0.0003659 ,
0.00018552, nan],
[0.00018602, 0.00036691, 0.00053812, ..., 0.0003659 ,
0.00018552, nan],
[ nan, nan, nan, ..., nan,
nan, nan]],
[[0.00018602, 0.0003669 , 0.00053812, ..., 0.00036589,
0.00018552, nan],
[0.00018602, 0.0003669 , 0.00053812, ..., 0.00036589,
0.00018552, nan],
[0.00018602, 0.0003669 , 0.00053812, ..., 0.00036589,
0.00018552, nan],
[ nan, nan, nan, ..., nan,
nan, nan]],
[[0.00018602, 0.00036689, 0.00053808, ..., 0.00036588,
0.00018552, nan],
[0.00018602, 0.00036689, 0.00053808, ..., 0.00036588,
0.00018552, nan],
[0.00018602, 0.00036689, 0.00053808, ..., 0.00036588,
0.00018552, nan],
[ nan, nan, nan, ..., nan,
nan, nan]],
...,
[[0.00018613, 0.00036707, 0.00053828, ..., 0.0003656 ,
0.00018537, nan],
[0.00018613, 0.00036707, 0.00053828, ..., 0.0003656 ,
0.00018537, nan],
[0.00018613, 0.00036707, 0.00053828, ..., 0.0003656 ,
0.00018537, nan],
[ nan, nan, nan, ..., nan,
nan, nan]],
[[0.00018642, 0.0003676 , 0.000539 , ..., 0.00036512,
0.00018511, nan],
[0.00018642, 0.0003676 , 0.000539 , ..., 0.00036512,
0.00018511, nan],
[0.00018642, 0.0003676 , 0.000539 , ..., 0.00036512,
0.00018511, nan],
[ nan, nan, nan, ..., nan,
nan, nan]],
[[0.0001875 , 0.00036966, 0.00054188, ..., 0.0003638 ,
0.0001844 , nan],
[0.0001875 , 0.00036966, 0.00054188, ..., 0.0003638 ,
0.0001844 , nan],
[0.0001875 , 0.00036966, 0.00054188, ..., 0.0003638 ,
0.0001844 , nan],
[ nan, nan, nan, ..., nan,
nan, nan]]])
Note that this is just a numpy array - if we want to make part of the Dataset we can just assign coordinates
with xr.open_dataset('example.snapshot.nc') as ds:
ds = ds.isel(Time=2)
ds['rough_speed'] = (('zt', 'yt', 'xt'), abs_speed)
display(ds.rough_speed)
<xarray.DataArray 'rough_speed' (zt: 90, yt: 4, xt: 200)> 0.000186 0.0003669 0.0005381 0.0006959 0.0008377 ... nan nan nan nan nan Coordinates: (4)
- zt: 90
- yt: 4
- xt: 200
- 0.000186 0.0003669 0.0005381 0.0006959 0.0008377 ... nan nan nan nan
array([[[0.00018602, 0.00036691, 0.00053812, ..., 0.0003659 , 0.00018552, nan], [0.00018602, 0.00036691, 0.00053812, ..., 0.0003659 , 0.00018552, nan], [0.00018602, 0.00036691, 0.00053812, ..., 0.0003659 , 0.00018552, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00018602, 0.0003669 , 0.00053812, ..., 0.00036589, 0.00018552, nan], [0.00018602, 0.0003669 , 0.00053812, ..., 0.00036589, 0.00018552, nan], [0.00018602, 0.0003669 , 0.00053812, ..., 0.00036589, 0.00018552, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.00018602, 0.00036689, 0.00053808, ..., 0.00036588, 0.00018552, nan], ... [ nan, nan, nan, ..., nan, nan, nan]], [[0.00018642, 0.0003676 , 0.000539 , ..., 0.00036512, 0.00018511, nan], [0.00018642, 0.0003676 , 0.000539 , ..., 0.00036512, 0.00018511, nan], [0.00018642, 0.0003676 , 0.000539 , ..., 0.00036512, 0.00018511, nan], [ nan, nan, nan, ..., nan, nan, nan]], [[0.0001875 , 0.00036966, 0.00054188, ..., 0.0003638 , 0.0001844 , nan], [0.0001875 , 0.00036966, 0.00054188, ..., 0.0003638 , 0.0001844 , nan], [0.0001875 , 0.00036966, 0.00054188, ..., 0.0003638 , 0.0001844 , nan], [ nan, nan, nan, ..., nan, nan, nan]]])
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- yt(yt)float64-0.5 0.5 1.5 2.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array([-0.5, 0.5, 1.5, 2.5])
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- Time()timedelta64[ns]03:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array(10800000000000, dtype='timedelta64[ns]')
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- ytPandasIndex
PandasIndex(Float64Index([-0.5, 0.5, 1.5, 2.5], dtype='float64', name='yt'))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
For fun, lets compare the two estimates along one slice in y:
with xr.open_dataset('example.snapshot.nc') as ds:
ds = ds.isel(Time=2)
ds['vcenter'] = ds.v.rolling(yu=2).mean().swap_dims({'yu':'yt'})
ds['ucenter'] = ds.u.rolling(xu=2).mean().swap_dims({'xu':'xt'})
ds['abs_speed'] = (ds.ucenter**2 + ds.vcenter**2)**(0.5)
ds['rough_speed'] = (('zt', 'yt', 'xt'), abs_speed)
ds = ds.isel(yt=1, yu=1)
fig, axs = plt.subplots(1, 3, sharex=True, sharey=True,
layout='constrained', subplot_kw={'facecolor': '0.7'},
figsize=(6, 4))
pc = axs[0].pcolormesh(ds.abs_speed, clim=[-1, 1], cmap='RdBu_r')
fig.colorbar(pc, extend='both', shrink=0.6, orientation='horizontal')
axs[0].set_title('speed: centered')
pc = axs[1].pcolormesh(ds.rough_speed, clim=[-1, 1], cmap='RdBu_r')
fig.colorbar(pc, extend='both', shrink=0.6, orientation='horizontal')
axs[1].set_title('speed: rough')
pc = axs[2].pcolormesh(ds.rough_speed - ds.abs_speed, clim=[-1/10, 1/10], cmap='RdBu_r')
fig.colorbar(pc, extend='both', shrink=0.6, orientation='horizontal')
axs[2].set_title('speed: difference')
axs[2].set_xlim([75, 125])
axs[2].set_ylim([20, 100])
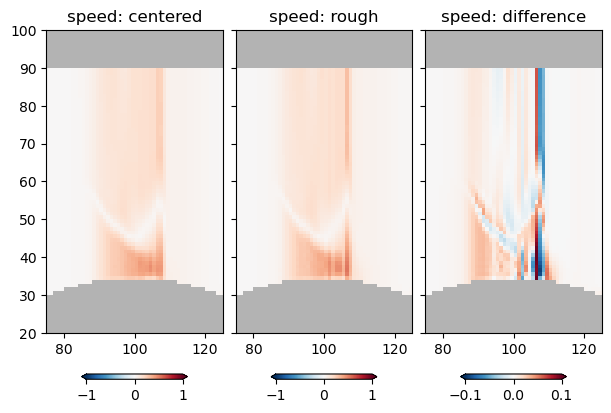
So there is some difference, but its maybe only noticable where there are sharp, poorly resolved parts of the velocity field.
Dealing with time#
You would think time would be straight forward - it rarely is.
Veros stores time in the Netcdf files in floating-point days since the start of the simulation. If you look at the default time values returned by xarray, it converts them to nanoseconds. I deal with this a few ways. Simplest is to convert back to days or hours:
with xr.open_dataset('example.snapshot.nc') as ds:
print(ds.Time.values)
ds['Time'] = ds.Time / np.timedelta64(1, 'h')
print('Hours', ds.Time.values)
with xr.open_dataset('example.snapshot.nc') as ds:
ds['Time'] = ds.Time / np.timedelta64(1, 'D')
print('Days', ds.Time.values)
[ 3600000028800 7199999971200 10800000000000 14400000028800
17999999971200 21600000000000]
Hours [1.00000001 1.99999999 3. 4.00000001 4.99999999 6. ]
Days [0.04166667 0.08333333 0.125 0.16666667 0.20833333 0.25 ]
This will almost always be appropriate for our needs. However, xarray forcing to nanoseconds has a big drawback for some of our longer simulations - after 292 years we run out of nanoseconds, and the numbers wrap! To get around this, we just use decode_timedelta=False
. Note that the resulting Time is now a float and has units “days”.
with xr.open_dataset('example.snapshot.nc', decode_timedelta=False) as ds:
display(ds.Time)
display(ds.Time.attrs)
<xarray.DataArray 'Time' (Time: 6)> 0.04167 0.08333 0.125 0.1667 0.2083 0.25 Coordinates: (1) Attributes: (3)
- Time: 6
- 0.04167 0.08333 0.125 0.1667 0.2083 0.25
array([0.041667, 0.083333, 0.125 , 0.166667, 0.208333, 0.25 ])
- Time(Time)float640.04167 0.08333 ... 0.2083 0.25
- long_name :
- Time
- units :
- days
- time_origin :
- 01-JAN-1900 00:00:00
array([0.041667, 0.083333, 0.125 , 0.166667, 0.208333, 0.25 ])
- TimePandasIndex
PandasIndex(Float64Index([0.041666666666666664, 0.08333333333333333, 0.125, 0.16666666666666666, 0.20833333333333331, 0.25], dtype='float64', name='Time'))
- long_name :
- Time
- units :
- days
- time_origin :
- 01-JAN-1900 00:00:00
{'long_name': 'Time', 'units': 'days', 'time_origin': '01-JAN-1900 00:00:00'}
Plotting and secondary calculations with data#
We saw an example of plotting data above. Ideally you have been exposed to data presentation techniques in other classes.
If you are using xarray you will likely use Matplotlib for data visualization. As in the example above, this usually consists of taking a slice of data and plotting with contour
or pcolormesh
. As an example that gets used a lot, lets use pcolormesh for velocity, and contour the temperature field over top:
Raw matplotlib:#
with xr.open_dataset('example.snapshot.nc') as ds:
# choose a time slice, and one value of yu and yt:
ds = ds.isel(Time=5, yu=1, yt=1)
fig, ax = plt.subplots(layout='constrained', figsize=(5, 3))
#make the background grey
ax.set_facecolor('0.5')
pc = ax.pcolormesh(ds.xt, ds.zw, ds.u[1:, 1:], clim=[-1, 1], cmap='RdBu_r')
ax.contour(ds.xt, ds.zt, ds.temp, levels=np.arange(10, 20, 2), colors='k', linewidths=0.4)
# label:
ax.set_xlabel('X [km]')
ax.set_ylabel('z [m]')
# make a colorbar
fig.colorbar(pc, shrink=0.6, extend='both')
# convert time to hours:
hours = int(ds.Time.values/ np.timedelta64(1, 'h'))
ax.set_title(f'Time = {hours}:00')
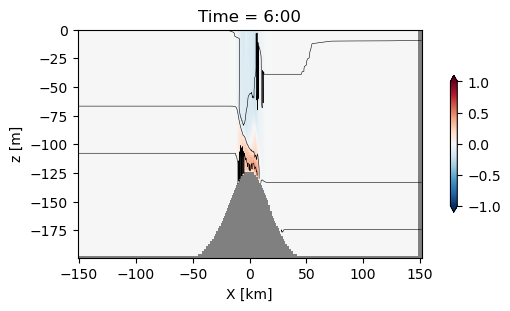
Sometimes it is nice to do lots of time slices, which is relatively straight forward. Note that to do this in a loop we use enumerate
to get the index of the time
we are on:
with xr.open_dataset('example.snapshot.nc') as ds0:
# choose a time slice, and one value of yu and yt:
ds0 = ds0.isel(yu=1, yt=1)
fig, axs = plt.subplots(2, 3, layout='constrained',
subplot_kw={'facecolor':'0.7'},
sharex=True, sharey=True)
# range(0, 6) returns 0, 1, 2,...5 eg the first 6 slices of the simulation.
# enumerate() is useful if your time slices are not integers, so
# nn, let = enumerate(['a', 'b', 'c']) returns nn=0, let='a'; nn=1, let='b';
# nn=2, let='c':
for nn, time in enumerate(range(0, 6)):
# choose time slice by index:
ds = ds0.isel(Time=time)
# choose the nn-th axes:
ax = axs.flat[nn]
#make the background grey
ax.set_facecolor('0.5')
pc = ax.pcolormesh(ds.xt, ds.zw, ds.u[1:, 1:], clim=[-1, 1], cmap='RdBu_r')
ax.contour(ds.xt, ds.zt, ds.temp, levels=np.arange(10, 20, 2), colors='k', linewidths=0.4)
# convert Time in nanoseconds to floating ploint hours:
hours = ds.Time.values/ np.timedelta64(1, 'h')
ax.set_title(f'{hours:1.2f}', fontsize='medium', loc='left')
# label the outside axes:
axs[0, 0].set_ylabel('z [m]')
axs[1, 2].set_xlabel('x [km]')
# make a colorbar for all at once:
fig.colorbar(pc, ax=axs, shrink=0.4, extend='both')
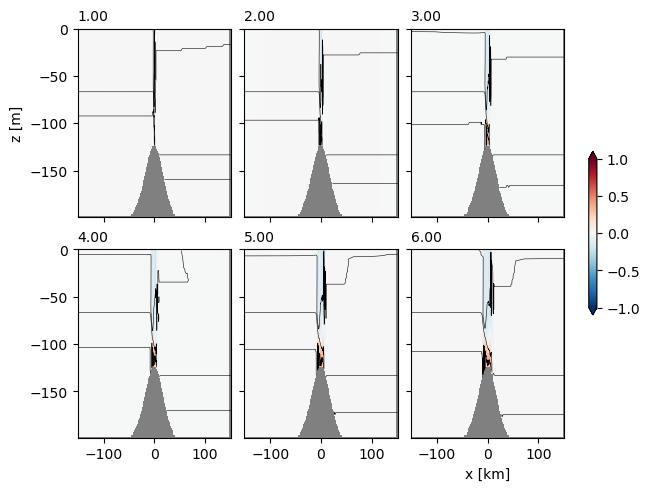
Xarray plotting helpers#
Xarray have some short cuts for plotting data. These can work well, particularly for a quick look, but lack some of the flexibility of the Matplotlib plotting:
with xr.open_dataset('example.snapshot.nc') as ds:
# choose a time slice, and one value of yu and yt:
ds = ds.isel(Time=5, yu=1, yt=1)
fig, ax = plt.subplots(layout='constrained', figsize=(5, 3))
#make the background grey
ax.set_facecolor('0.5')
ds.u.plot.pcolormesh(clim=[-1, 1])
ds.temp.plot.contour(levels=np.arange(10, 20, 2), colors='k', linewidths=0.4)
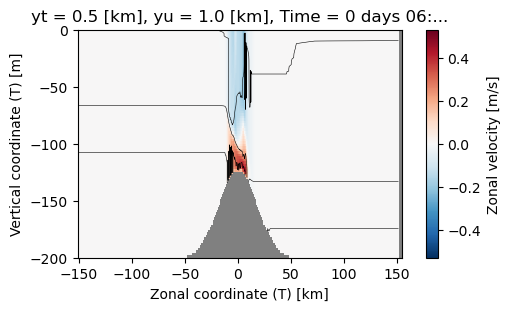
This is perfectly acceptable; note how xarray makes the labels for us, which is quite convenient. You probably would not want to publish a plot like this, but could be fine.
Note a trick is that if you don’t like ‘Zonal coordinate (T) [km]’, you can go ahead and change the attributes - its a bit silly to do so here, but if you develop a processing script for your xarray data you could easily do this every time you load a data set.
with xr.open_dataset('example.snapshot.nc') as ds:
# choose a time slice, and one value of yu and yt:
ds = ds.isel(Time=5, yu=1, yt=1)
ds.xu.attrs['long_name'] = '$X_u$'
ds.xt.attrs['long_name'] = '$X_t$'
ds.zt.attrs['long_name'] = '$z_t$'
ds.zw.attrs['long_name'] = '$z_w$'
ds.u.attrs['long_name'] = 'U'
ds.u.attrs['units'] = '$m\,s^{-1}$'
fig, ax = plt.subplots(layout='constrained', figsize=(5, 3))
#make the background grey
ax.set_facecolor('0.5')
ds.u.plot.pcolormesh(clim=[-1, 1])
ds.temp.plot.contour(levels=np.arange(10, 20, 2), colors='k', linewidths=0.4)
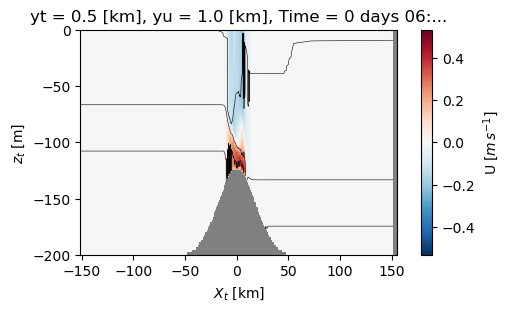
Integration#
Often we will want to integrate something. Suppose for the above we want to get an estimate of the heat content:
where \(\rho = 1000 \ \mathrm{kg\,m^{-3}}\) is the density of water, \(c_p = 4000\ \mathrm{J/(kg K)}\) is the heat capacity of seawater, and \(Q\) has units of \(J\,m^{-2}\) and is a density of heat content per area in the horizontal direction.
with xr.open_dataset('example.snapshot.nc') as ds:
# choose a time slice, and one value of yu and yt:
ds = ds.isel(Time=5, yu=1, yt=1)
rho = 1000 # kg/m^3
cp = 4000 # J / (kg K)
heat = (ds.temp+273).integrate(coord='zt') * cp * rho
display(heat)
fig, ax = plt.subplots()
ax.plot(heat.xt, heat)
<xarray.DataArray 'temp' (xt: 200)> 2.249e+11 2.249e+11 2.249e+11 2.249e+11 ... 2.26e+11 2.26e+11 2.26e+11 2.26e+11 Coordinates: (4)
- xt: 200
- 2.249e+11 2.249e+11 2.249e+11 2.249e+11 ... 2.26e+11 2.26e+11 2.26e+11
array([2.24932734e+11, 2.24932734e+11, 2.24932734e+11, 2.24932734e+11, 2.24932734e+11, 2.24932734e+11, 2.24932734e+11, 2.24932734e+11, 2.24932733e+11, 2.24932733e+11, 2.24932733e+11, 2.24932733e+11, 2.24932733e+11, 2.24932733e+11, 2.24932733e+11, 2.24932733e+11, 2.24932732e+11, 2.24932732e+11, 2.24932732e+11, 2.24932732e+11, 2.24932732e+11, 2.24932732e+11, 2.24932732e+11, 2.24932733e+11, 2.24932733e+11, 2.24932734e+11, 2.24932735e+11, 2.24932737e+11, 2.24932737e+11, 2.24932736e+11, 2.24932736e+11, 2.24932747e+11, 2.24932733e+11, 2.24932735e+11, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, ... nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, 2.26000416e+11, 2.26000412e+11, 2.26000380e+11, 2.26000398e+11, 2.26000394e+11, 2.26000387e+11, 2.26000385e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000387e+11, 2.26000387e+11, 2.26000387e+11, 2.26000387e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000385e+11, 2.26000385e+11, 2.26000384e+11, 2.26000383e+11, 2.26000383e+11, 2.26000383e+11])
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- yt()float640.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array(0.5)
- yu()float641.0
- long_name :
- Meridional coordinate (U)
- units :
- km
array(1.)
- Time()timedelta64[ns]06:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array(21600000000000, dtype='timedelta64[ns]')
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
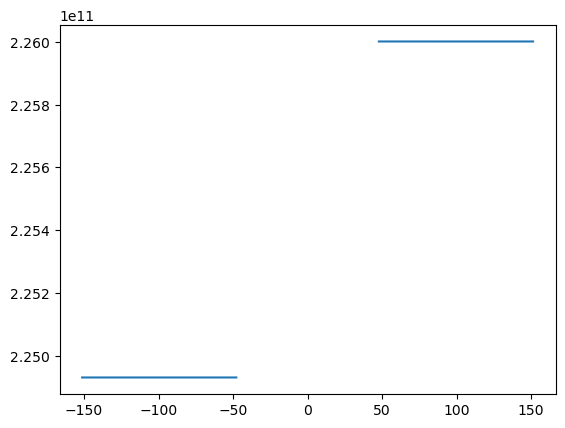
So, we see above that this probably worked, but that there is an error in that there are many nan
values that occured because we integrated into the topography. Rather than trying to do something fancy, a reasonable approach is just to replace the nan
in the original temp
variable by zeros:
with xr.open_dataset('example.snapshot.nc') as ds:
# choose a time slice, and one value of yu and yt:
ds = ds.isel(Time=5, yu=1, yt=1)
rho = 1000 # kg/m^3
cp = 4000 # J / (kg K)
temp=(ds.temp+273).fillna(0)
heat = temp.integrate(coord='zt') * cp * rho
display(heat)
<xarray.DataArray 'temp' (xt: 200)> 2.249e+11 2.249e+11 2.249e+11 2.249e+11 ... 2.26e+11 2.26e+11 2.26e+11 2.26e+11 Coordinates: (4)
- xt: 200
- 2.249e+11 2.249e+11 2.249e+11 2.249e+11 ... 2.26e+11 2.26e+11 2.26e+11
array([2.24932734e+11, 2.24932734e+11, 2.24932734e+11, 2.24932734e+11, 2.24932734e+11, 2.24932734e+11, 2.24932734e+11, 2.24932734e+11, 2.24932733e+11, 2.24932733e+11, 2.24932733e+11, 2.24932733e+11, 2.24932733e+11, 2.24932733e+11, 2.24932733e+11, 2.24932733e+11, 2.24932732e+11, 2.24932732e+11, 2.24932732e+11, 2.24932732e+11, 2.24932732e+11, 2.24932732e+11, 2.24932732e+11, 2.24932733e+11, 2.24932733e+11, 2.24932734e+11, 2.24932735e+11, 2.24932737e+11, 2.24932737e+11, 2.24932736e+11, 2.24932736e+11, 2.24932747e+11, 2.24932733e+11, 2.24932735e+11, 2.23674935e+11, 2.23674982e+11, 2.21159381e+11, 2.21159405e+11, 2.18643824e+11, 2.16128265e+11, 2.13612723e+11, 2.11097170e+11, 2.08581592e+11, 2.06066013e+11, 2.03550470e+11, 2.01034939e+11, 1.98519392e+11, 1.96003814e+11, 1.93488239e+11, 1.93488246e+11, 1.90972703e+11, 1.88457152e+11, 1.85941588e+11, 1.83426008e+11, 1.80910469e+11, 1.78394896e+11, 1.78394959e+11, 1.75879461e+11, 1.73363961e+11, 1.73364088e+11, 1.70848648e+11, 1.68333221e+11, 1.68333452e+11, 1.65818184e+11, 1.65818607e+11, 1.63303628e+11, 1.63304495e+11, 1.60790210e+11, 1.60792229e+11, 1.58280079e+11, 1.58286111e+11, 1.55781348e+11, 1.55799199e+11, 1.53310805e+11, 1.53345550e+11, 1.50870045e+11, 1.50905355e+11, 1.50940905e+11, 1.48455814e+11, 1.48484302e+11, ... 1.49019838e+11, 1.49077589e+11, 1.51649050e+11, 1.51675826e+11, 1.51690772e+11, 1.54228278e+11, 1.54239414e+11, 1.56766966e+11, 1.56778578e+11, 1.59304021e+11, 1.59313781e+11, 1.61835252e+11, 1.61840955e+11, 1.64359481e+11, 1.64362582e+11, 1.66879689e+11, 1.66881363e+11, 1.69397811e+11, 1.69398685e+11, 1.71914652e+11, 1.74430591e+11, 1.74430953e+11, 1.76946691e+11, 1.79462434e+11, 1.79462635e+11, 1.81978284e+11, 1.84493877e+11, 1.87009467e+11, 1.89525036e+11, 1.92040589e+11, 1.94556150e+11, 1.94556163e+11, 1.97071637e+11, 1.99587192e+11, 2.02102806e+11, 2.04618417e+11, 2.07133937e+11, 2.09649417e+11, 2.12164941e+11, 2.14680499e+11, 2.17196071e+11, 2.19711612e+11, 2.22227102e+11, 2.22227143e+11, 2.24742602e+11, 2.24742687e+11, 2.26000416e+11, 2.26000412e+11, 2.26000380e+11, 2.26000398e+11, 2.26000394e+11, 2.26000387e+11, 2.26000385e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000387e+11, 2.26000387e+11, 2.26000387e+11, 2.26000387e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000386e+11, 2.26000385e+11, 2.26000385e+11, 2.26000384e+11, 2.26000383e+11, 2.26000383e+11, 2.26000383e+11])
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- yt()float640.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array(0.5)
- yu()float641.0
- long_name :
- Meridional coordinate (U)
- units :
- km
array(1.)
- Time()timedelta64[ns]06:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array(21600000000000, dtype='timedelta64[ns]')
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
fig, ax = plt.subplots()
ax.plot(heat.xt, heat)
[<matplotlib.lines.Line2D at 0x161156f70>]
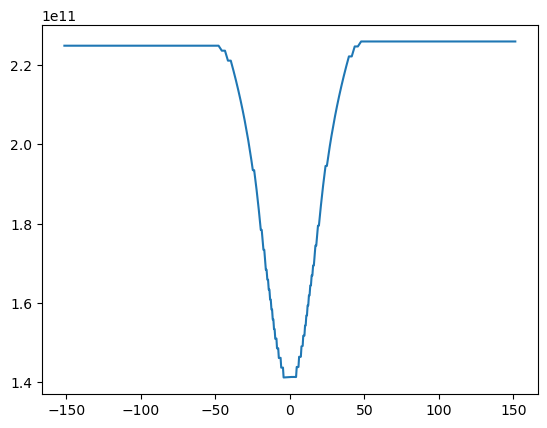
Differentiation#
Xarray also has a differentiation method. The value \(-\frac{1}{\rho}dP/dx\) is used quite often. Note that in Veros, the density is broken into an interior component, p_hydro
and a component due to the surface tilt psi
. The dynamical quantity is really P/\rho
so Veros stores the variables in these units (\(\mathrm{m^2\,s^{-2}}\)). Note that \(-\frac{1}{\rho}dP/dx\) has units of acceleration and is the pressure gradient force.
with xr.open_dataset('example.snapshot.nc') as ds:
# choose a time slice, and one value of yu and yt:
ds = ds.isel(Time=5, yu=1, yt=1)
dpdx = -ds.p_hydro.differentiate(coord='xt')
dpsidx = -ds.psi.differentiate(coord='xt')
display(dpdx)
fig, axs = plt.subplots(2, 1, layout='constrained', sharex=True, sharey=True)
ax = axs[0]
ax.set_facecolor('0.5')
dpdx.plot.pcolormesh(ax=axs[0], vmin=-0.05, vmax=0.05, cmap='RdBu_r')
ax.set_title('internal pressure gradient')
ax = axs[1]
ax.set_facecolor('0.5')
(dpdx+dpsidx).plot.pcolormesh(ax=axs[1], vmin=-0.05, vmax=0.05, cmap='RdBu_r')
ax.set_title('internal pressure gradient and external')
<xarray.DataArray 'p_hydro' (zt: 90, xt: 200)> -2.219e-08 -2.537e-08 -3.084e-08 -2.792e-08 ... 6.678e-08 4.671e-08 3.327e-08 Coordinates: (5)
- zt: 90
- xt: 200
- -2.219e-08 -2.537e-08 -3.084e-08 ... 6.678e-08 4.671e-08 3.327e-08
array([[-2.21863383e-08, -2.53675649e-08, -3.08425578e-08, ..., 2.91363289e-09, 3.01827896e-09, 2.18905716e-09], [-2.21863383e-08, -2.53675649e-08, -3.08425578e-08, ..., 2.91363289e-09, 3.01827985e-09, 2.18905716e-09], [-2.21863383e-08, -2.53675640e-08, -3.08425578e-08, ..., 2.91363378e-09, 3.01827985e-09, 2.18905716e-09], ..., [-3.82663027e-08, -5.06896659e-08, -7.01157333e-08, ..., 1.87898054e-07, 1.29826336e-07, 9.20596128e-08], [-2.30228281e-08, -3.05083850e-08, -4.22272395e-08, ..., 1.60503364e-07, 1.11437528e-07, 7.91627391e-08], [-7.69097280e-09, -1.01945366e-08, -1.41176338e-08, ..., 6.67848989e-08, 4.67066305e-08, 3.32705408e-08]])
- xt(xt)float64-151.3 -147.8 ... 147.8 151.3
- long_name :
- Zonal coordinate (T)
- units :
- km
array([-151.317528, -147.84833 , -144.386624, -140.925949, -137.473937, -134.024281, -130.584789, -127.149353, -123.725998, -120.308864, -116.906251, -113.512602, -110.136554, -106.772921, -103.430743, -100.105274, -96.806029, -93.52877 , -90.283551, -87.066694, -83.888832, -80.746875, -77.652035, -74.601777, -71.607826, -68.668094, -65.794658, -62.98566 , -60.253259, -57.5955 , -55.024242, -52.537006, -50.144894, -47.844439, -45.64553 , -43.543288, -41.546028, -39.647184, -37.853322, -36.156127, -34.560478, -33.056486, -31.647619, -30.322773, -29.084428, -27.920725, -26.833619, -25.810951, -24.854579, -23.952426, -23.106581, -22.305317, -21.55117 , -20.832928, -20.153681, -19.502796, -18.883951, -18.287092, -17.716457, -17.162531, -16.630061, -16.110005, -15.60755 , -15.114059, -14.635089, -14.16234 , -13.701673, -13.245061, -12.798612, -12.354521, -11.919091, -11.484694, -11.057788, -10.630883, -10.210561, -9.789439, -9.410561, -8.989439, -8.610561, -8.189439, -7.810561, -7.389439, -7.010561, -6.589439, -6.210561, -5.789439, -5.410561, -4.989439, -4.610561, -4.189439, -3.810561, -3.389439, -3.010561, -2.589439, -2.210561, -1.789439, -1.410561, -0.989439, -0.610561, -0.189439, 0.189439, 0.610561, 0.989439, 1.410561, 1.789439, 2.210561, 2.589439, 3.010561, 3.389439, 3.810561, 4.189439, 4.610561, 4.989439, 5.410561, 5.789439, 6.210561, 6.589439, 7.010561, 7.389439, 7.810561, 8.189439, 8.610561, 8.989439, 9.410561, 9.789439, 10.210561, 10.630883, 11.057788, 11.484694, 11.919091, 12.354521, 12.798612, 13.245061, 13.701673, 14.16234 , 14.635089, 15.114059, 15.60755 , 16.110005, 16.630061, 17.162531, 17.716457, 18.287092, 18.883951, 19.502796, 20.153681, 20.832928, 21.55117 , 22.305317, 23.106581, 23.952426, 24.854579, 25.810951, 26.833619, 27.920725, 29.084428, 30.322773, 31.647619, 33.056486, 34.560478, 36.156127, 37.853322, 39.647184, 41.546028, 43.543288, 45.64553 , 47.844439, 50.144894, 52.537006, 55.024242, 57.5955 , 60.253259, 62.98566 , 65.794658, 68.668094, 71.607826, 74.601777, 77.652035, 80.746875, 83.888832, 87.066694, 90.283551, 93.52877 , 96.806029, 100.105274, 103.430743, 106.772921, 110.136554, 113.512602, 116.906251, 120.308864, 123.725998, 127.149353, 130.584789, 134.024281, 137.473937, 140.925949, 144.386624, 147.84833 , 151.317528])
- yt()float640.5
- long_name :
- Meridional coordinate (T)
- units :
- km
array(0.5)
- yu()float641.0
- long_name :
- Meridional coordinate (U)
- units :
- km
array(1.)
- zt(zt)float64-198.9 -196.7 ... -3.333 -1.111
- long_name :
- Vertical coordinate (T)
- units :
- m
- positive :
- up
array([-198.888889, -196.666667, -194.444444, -192.222222, -190. , -187.777778, -185.555556, -183.333333, -181.111111, -178.888889, -176.666667, -174.444444, -172.222222, -170. , -167.777778, -165.555556, -163.333333, -161.111111, -158.888889, -156.666667, -154.444444, -152.222222, -150. , -147.777778, -145.555556, -143.333333, -141.111111, -138.888889, -136.666667, -134.444444, -132.222222, -130. , -127.777778, -125.555556, -123.333333, -121.111111, -118.888889, -116.666667, -114.444444, -112.222222, -110. , -107.777778, -105.555556, -103.333333, -101.111111, -98.888889, -96.666667, -94.444444, -92.222222, -90. , -87.777778, -85.555556, -83.333333, -81.111111, -78.888889, -76.666667, -74.444444, -72.222222, -70. , -67.777778, -65.555556, -63.333333, -61.111111, -58.888889, -56.666667, -54.444444, -52.222222, -50. , -47.777778, -45.555556, -43.333333, -41.111111, -38.888889, -36.666667, -34.444444, -32.222222, -30. , -27.777778, -25.555556, -23.333333, -21.111111, -18.888889, -16.666667, -14.444444, -12.222222, -10. , -7.777778, -5.555556, -3.333333, -1.111111])
- Time()timedelta64[ns]06:00:00
- long_name :
- Time
- time_origin :
- 01-JAN-1900 00:00:00
array(21600000000000, dtype='timedelta64[ns]')
- xtPandasIndex
PandasIndex(Float64Index([-151.31752835206134, -147.84832992570608, -144.3866239045746, -140.92594922692336, -137.4739367427506, -134.0242813779343, -130.5847894272687, -127.14935274843452, -123.72599817615092, -120.30886389132668, ... 120.3088638913266, 123.72599817615095, 127.14935274843441, 130.58478942726867, 134.02428137793416, 137.4739367427506, 140.92594922692328, 144.38662390457466, 147.84832992570603, 151.3175283520614], dtype='float64', name='xt', length=200))
- ztPandasIndex
PandasIndex(Float64Index([-198.88888888888926, -196.66666666666703, -194.4444444444448, -192.2222222222226, -190.00000000000037, -187.77777777777814, -185.5555555555559, -183.3333333333337, -181.11111111111148, -178.88888888888926, -176.66666666666703, -174.4444444444448, -172.2222222222226, -170.00000000000037, -167.77777777777814, -165.55555555555594, -163.3333333333337, -161.11111111111148, -158.88888888888926, -156.66666666666703, -154.4444444444448, -152.2222222222226, -150.00000000000037, -147.77777777777817, -145.55555555555594, -143.3333333333337, -141.11111111111148, -138.88888888888926, -136.66666666666703, -134.44444444444483, -132.2222222222226, -130.00000000000037, -127.77777777777814, -125.55555555555591, -123.33333333333368, -121.11111111111146, -118.88888888888923, -116.666666666667, -114.44444444444477, -112.22222222222254, -110.00000000000031, -107.77777777777808, -105.55555555555586, -103.33333333333363, -101.1111111111114, -98.88888888888917, -96.66666666666694, -94.44444444444471, -92.22222222222248, -90.00000000000026, -87.77777777777803, -85.5555555555558, -83.33333333333357, -81.11111111111134, -78.88888888888911, -76.66666666666688, -74.44444444444466, -72.22222222222243, -70.0000000000002, -67.77777777777797, -65.55555555555574, -63.33333333333351, -61.111111111111285, -58.888888888889056, -56.66666666666683, -54.4444444444446, -52.22222222222237, -50.00000000000014, -47.777777777777914, -45.555555555555685, -43.33333333333346, -41.11111111111123, -38.888888888889, -36.66666666666677, -34.44444444444454, -32.222222222222314, -30.000000000000085, -27.777777777777857, -25.555555555555628, -23.3333333333334, -21.11111111111117, -18.888888888888943, -16.666666666666714, -14.444444444444485, -12.222222222222257, -10.000000000000028, -7.7777777777778, -5.555555555555571, -3.333333333333343, -1.1111111111111143], dtype='float64', name='zt'))
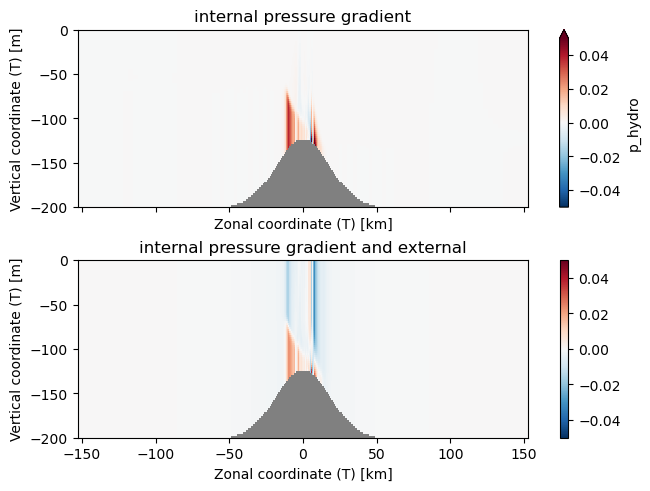
Note the stripe in the plot above are due to discretiztion errors, and are a fundamental limitation of working on a grid with numerical methods.
Final notes#
Sometimes dealing with these data sets is frustrating, and takes a bit of experimentation to get things to work. If you have trouble, ask a class mate, or the instructor - don’t bang your head against a wall for too long.